JavaScript is a powerful client side programming language used by every website. JS developers heavily rely on arrays to store, transfer and read data. While working with JS arrays, often you may need to compare two arrays. Typically, developers use comparison operators such as == to compare arrays but it does not give the correct result. In this article, we will learn how to compare arrays in JavaScript.
How to Compare Arrays in JavaScript
Let us say you have two arrays.
var a1 = [1,2,3]; var a2 = [1,2,3];
As you can see, they are equal but if you try to compare them using comparison operator, you will get an unexpected result.
console.log(a1==a2); // Returns false
So how do we compare two arrays properly? There are a couple of ways to do this.
1. Using JSON Stringify
One of the simplest ways to compare two arrays is to convert them into JSON strings and compare the two strings for equality. This works because comparison operators work on strings and numbers. JSON string is treated just as any other string.
Here is an example.
console.log(JSON.stringify(a1)==JSON.stringify(a2)); // Returns true
2. Compare Each Element
Another way is to loop through each array and compare each element of one array to its corresponding element in the other array. The following method works for scalar arrays, that is, arrays of numbers, strings, objects, and objects by references and function by references.
First we compare the lengths of two arrays.
a1.length === a2.length
Next we compare each element of first array with its corresponding element from the other array. We use every() function to check equality between each pair or elements. Every() function executes a function for each element of an array and returns true only if results of all function calls are true. Else it returns false.
a1.every(function(value, index) { return value === a2[index]})
The above command will return true only if both arrays are identical, else it will return false. We can combine both the conditions using && operator as shown below.
a1.length === a2.length && a1.every(function(value, index) { return value === a2[index]})
The above code assumes that both your arrays have the same sort order. Sometimes both your arrays may contain the same elements but in different order. If you want to treat such arrays as equal then you need to sort the arrays before comparing. For example, if you use the above code on the following arrays, you will get result as false.
a1 = [2,3,1,4]; a2 = [1,2,3,4];
But as you can see both the arrays contain same elements but in different orders. If you want to treat the above arrays also as equal then you need to modify your code to sort the arrays before comparison.
const a2Sorted = a2.slice().sort(); a1.length === a2.length && a1.slice().sort().every(function(value, index) { return value === a2Sorted[index]; });
When you are comparing arrays, if you are particular that both arrays have the same order of elements, use the first code above, else you can use the second one.
In this article, we have learnt a couple of simple ways to check if two arrays are equal in JavaScript. If you are comparing each element of an array with the other, make sure to first check if the lengths of both arrays are same or not.
Also read:
How to Add Days to Date in JavaScript
How to Compare Two Dates Using JavaScript
How to Get Nested Object Keys in JavaScript
How to Overwrite Input File in Awk
How to Read Command Line Arguments in NodeJS
Related posts:
How to Detect Tab/Browser Closing in JavaScript
How to Render HTML in TextArea
How to Validate Decimal Number in JavaScript
How to Check if Variable is Object in JS
How to Capitalize First Letter in JavaScript
JavaScript Round to 2 Decimal Places
How to Find Element Using XPath in JavaScript
How to Remove Duplicates from Array of Objects JavaScript
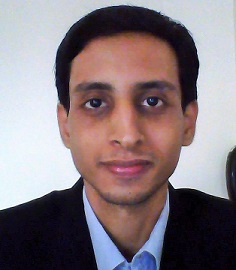
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.