JavaScript allows you to store data as key-value pairs called JS objects. Their structure is similar to JSON and even supports nesting, that is, using one object in another. Sometimes you may need to get keys from objects in JavaScript. In this article, we will learn how to get nested object keys in JavaScript.
How to Get Nested Object Keys in JavaScript
Let us say you have the following nested object in JavaScript.
let data = [ { name: "Apple", id: 1, alt: [{ name: "fruit1", description: "tbd1" }] }, { name: "Banana", id: 2, alt: [{ name: "fruit2", description: "tbd2" }] }, { name: "Blueberry", id: 3, alt: [{ name: "fruit3", description: "tbd3" }] } ];
Every JS object supports keys() function that returns an array of keys present in the first level of object. Here is its syntax.
let keys = Object.keys(yourObject);
You can access the nested object using indexes 0, 1, 2, etc. So data[0].alt[0] will access the first alt nested object. Now you can call the above mentioned keys() function to get a list of its keys.
Object.keys(data[0].alt[0])
The above command will return [‘name’, ‘description’] not necessarily in the same order.
If you want to get the value of a specific key, you can directly refer to it using its key name. Here is the command to get description value of first nested object.
data[0].alt[0].description
If you want to get an array of description value of all nested objects, use the following syntax.
data.map(obj => obj.alt[0].description)
In this article, we have learnt how to get nested object keys in JavaScript.
Also read:
How to Read Command Line Arguments in NodeJS
How to Change Element’s Class Name in JavaScript
JavaScript Round to 2 Decimal Places
How to Disable Right Click on Web Page Using JavaScript
How to Disable/Enable Input in jQuery
Related posts:
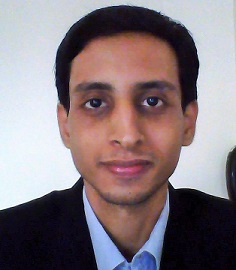
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.