Sometimes you may want to round numbers to at most 2 decimal places. In this article, we will learn how to round numbers to 2 decimal places using JavaScript.
Let us look at the following example. We have defined a variable where we add two numbers, and then we print the result.
num = 0.1 + 0.2 console.log(num) // 0.30000000000000004
When you run the above code, you will notice that the number of decimals in the result is unusually large. This is a typical JavaScript problem where the number of places in result is much more than those in original numbers used to calculate the number.
JavaScript Round to 2 Decimal Places
One of the most common ways to round a number to specific number of places such as 2 places is using Math.toFixed() function that allows you to specify the number of decimal places to be kept in result.
num = 0.1 + 0.2 num = num.toFixed(2) console.log(num) // 0.30
In the above example, you will see that the result has precisely 2 decimal places.
The above approach works with most numbers but it will always show 2 decimal places in the result, even when it is an integer. Here is an example.
num = 0.5 + 0.5 num = num.toFixed(2) console.log(num) // 1.00
If the above result is not desirable to you, then there is another way to round the number such that it shows at the most 2 decimal places, using Math.round() function. In this case, we basically multiply the number by 100 and call Math.round() function on the result. Further, we divide the same number by 100. Here is an example.
Math.round(num * 100) / 100
Here are some examples to illustrate how it works.
num = 0.5 + 0.5 num = Math.round(num * 100) / 100 console.log(num) // 1 num = 0.2 + 0.5 num = Math.round(num * 100) / 100 console.log(num) // 0.7 num = 0.51 + 0.53 num = Math.round(num * 100) / 100 console.log(num) // 1.04 num = 0.513 + 0.512 num = Math.round(num * 100) / 100 console.log(num) // 1.03
As you can see, this is a very neat way to round the numbers such that at most it will display up to 2 decimal places. The number of decimal places in output automatically changes to drop trailing zeroes.
Similarly, if you want to round to 3 decimal places, you need to multiply the number by 1000 and then call Math.round() function on it, and then divide it by 1000.
num = 0.5 + 0.5 num = Math.round(num * 1000) / 1000 console.log(num) // 1
In this article, we have learnt how to round to 2 decimal places using JavaScript.
Also read:
How to Disable Right Click on Web Page Using JavaScript
How to Disable/Enable Input in jQuery
How to Run 32-bit App in 64-bit Linux
How to Change File Extension of Multiple Files in Python
How to Change File Extension of Multiple Files in Linux
Related posts:
How to Sum Values of JS Object
How to Get Highlighted/Selected Text in JavaScript
How to Change Href Attribute of Link Using jQuery
How to Get HTML Element's Actual Width & Height
How to Call JS Function in IFrame from Parent Page
How to Find Element Using XPath in JavaScript
How to Convert Comma Separated String into JS Array
How to Get Array Intersection in JavaScript
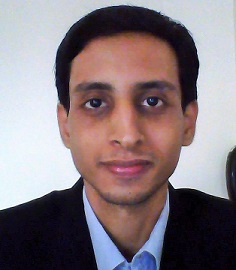
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.