JavaScript objects allow you to store data as key-value pairs in a compact manner. Often while using JS objects you may need to add up the values of all or some JS objects. It can be tedious to write code to iterate through each JS object and add it to the sum. It would be a lot easier if you created a function or prototype that does this for you. In this article, we will learn how to sum values of JS object.
How to Sum Values of JS Object
Let us say you have the following JS object.
sample = { 'a': 1 , 'b': 2 , 'c':3 };
We can create a prototype as shown below to easily sum all JS objects on our page.
const sum_object = obj => Object.values(obj).reduce((a, b) => a + b, 0);
In the above code, object.values() returns an array of property values of JS object, in the same order as that returned by a for loop. The reduce() function applies a function to an accumulator and each value of array to reduce it to a single value.
Once you have defined the above function, you can use it as shown below.
sumValues({a: 4, b: 6, c: 5, d: 0}); // returns 15
If the above code is not supported in your browser, or if you are looking for good old fashioned JS function, you can try the following code snippet instead.
function sum( obj ) { var sum = 0; for( var el in obj ) { if( obj.hasOwnProperty( el ) ) { sum += parseFloat( obj[el] ); } } return sum; }
The above function accepts a JS object as input argument. It initiates a sum variable to zero, this will hold the final as well as intermediate sum values. We use a for loop to iterate through the JS object. In each iteration, we first check if the object as a property, and if so, we add it to the sum. While adding the value we call parseFloat() function to convert the value into a number, just in case some of the numbers are stored as strings. You can customize this function as per your requirement.
Once you have defined the above function, you can call it as shown below.
var total = sum( sample ); console.log(total); // returns 15
In fact you can combine both the above approaches into one, as shown below, if you want. Here we create a function that uses reeduce() function to sum the object values.
function sum(obj) { return Object.keys(obj).reduce((sum,key)=>sum+parseFloat(obj[key]||0),0); } OR function sum(obj) { return Object.values(obj).reduce((a, b) => a + b, 0); }
In this article, we have learnt several different ways to sum JS objects. You can use any of them as per your needs.
Also read:
How to Access POST Form Fields in ExpressJS
How to Detect Scroll Direction in JavaScript
How to Split String With Multiple Delimiters in JS
How to Split String With Multiple Delimiters in Python
How to Clone Array in JavaScript
Related posts:
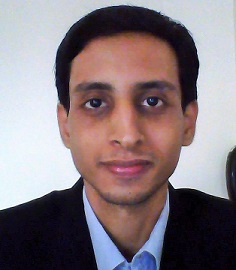
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.