Often JavaScript developers need to use dates as input or temporary data. While working with dates, it is advisable to first check if the input data is a valid date or not. Otherwise, JavaScript will give an error and terminate execution. So it is a good validation to check if a given date is valid or not in JavaScript, especially if you have user input. In this article, we will learn how to detect invalid date in JavaScript.
How to Detect Invalid Date in JavaScript
Here are the steps to test if a date is valid or not in JavaScript.
Let us say you have the following input data.
d='2022-10-02 23:30:00'
First we check if the variable was created using Date object or not using Object.prototype.toString.call(d) method. If the date is valid date object then when you invoke getTime() function on it, you will get its proper time value. Else you will get NaN.
So once you have determined if it is a date object, you can use isNaN() or getTime() or valueOf() function to determine if it is NaN or not.
isNaN(d)
If the result of above function is false, then the input value is date, else it is not a valid date. Putting it all together,
if (Object.prototype.toString.call(d) === "[object Date]") { // it is a date if (isNaN(d)) { // d.getTime() or d.valueOf() will also work // date object is not valid } else { // date object is valid } } else { // not a date object }
The above function takes care of pretty much all kinds of date inputs including windows, frames, and iframes. If you are not concerned about those inputs, you can use a shorter form of the above code.
function isValidDate(d) { return d instanceof Date && !isNaN(d); }
Please note the above code only validates if a given input variable is a proper date object or not. It does not tell if the input is a proper date or not. For example, it will return false if your input is a string like ‘foo’ since when you pass it to Date() constructor it returns NaN or ‘Invalid Date’. On the other hand it will return true even if you check Date(1) with the above code since Date(1) returns Jan 1, 1970 which is a valid date.
In the above article, we have learnt a couple of simple ways to determine if a date is valid or not in JavaScript. You can use this as a part of bigger function or validation module as per your requirement.
Also read:
How to Convert Date into Another Timezone in JavaScript
How to Compare Two Arrays in JavaScript
How to Add Days to Date in JavaScript
How to Compare Two Dates in JavaScript
How to Get Nested Objects Keys in JavaScript
Related posts:
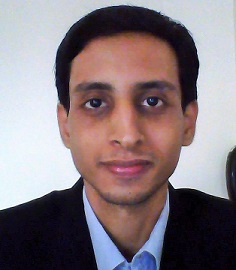
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.