JavaScript allows you to work with dates – create, modify and compare dates. But sometimes you may need to add days to a specific date in JavaScript. While there are many popular third-party JavaScript libraries such as Moment.js, you can also do this using plain JavaScript. In this article, we will learn how to add days to date in JavaScript.
How to Add Days to Date in JavaScript
Here are the steps to add days to date in JavaScript. Every date object in JavaScript supports adding dates using mathematical operators. It also supports a function setDate() where you can set it to a new date. For example, if you have a date object d1 and want to add n days to it, then you can do so with the following command to add n days to it. We simply call getDate() function on our starting date and add number of days using addition operator.
d1.getDate() + n
Please note, you cannot directly add days to a Date object, but you can do so to the result of getDate() function called on it. You can set another date d2 to the above value using setDate() function.
d1.setDate(d1.getDate() + days)
Putting together the above commands, we can create a JavaScript function to add n days to a given date d1.
function addDays(d1, days) { const d2 = new Date(Number(d1)) d2.setDate(d1.getDate() + days) return copy }
Here is how you can call it. Here is an example to add 10 days to today’s date.
const date = new Date(); const newDate = addDays(date, 10);
Update JavaScript Date by Adding Days
You can also directly update a given date by adding days to it.
const date = new Date(); date.setDate(date.getDate() + 10);
Add 1 Day to JavaScript Date
Similarly, here is the command to add a day to JavaScript date.
const date = new Date(); date.setDate(date.getDate() + 1);
Add Week to JavaScript Date
You can also add a 1 week to JavaScript date.
const date = new Date(); date.setDate(date.getDate() + 7);
You will also notice that in this method JavaScript correctly changes the month, if number of days to be added is more than the number of days left in present month. Here is an example to illustrate this.
const d1 = new Date('2019-04-14'); const d2 = addDays(myDate, 31); console.log(d2) // 2019-05-15
In the above example, the month has changed from April to May since we are adding 31 days to April 14, 2019.
In this article, we have learnt how to add days to date in JavaScript, and also covered some basic use cases for it. The key is to first create a Date object for your starting date, then add the number of days to the result of getDate() function called on your Date object. Optionally, you can store this new date value in another date using SetDate() function.
Also read:
How to Compare Two Dates in JavaScript
How to Get Nested Object Keys in JavaScript
How to Overwrite Input File in Awk
How to Read Command Line Arguments in NodeJS
How to Change Element’s Class Name in JavaScript
Related posts:
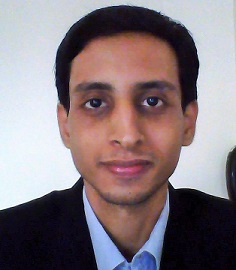
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.