MongoDB is a popular NoSQL database that organizes your data in the form of key-value pairs in documents. As a database administrator, you will need to often backup MongoDB database on your system. While there is an out-of-the-box command to backup MongoDB database, it is advisable to automate database backups using shell scripts and schedule them to run as a cronjob. It will save you a lot of hassle. In this article, we will learn how to create a shell script to backup MongoDB database in Linux.
Shell Script to Backup MongoDB Database
The basic command to backup MongoDB database is as follows
mongodump --host <hostname> --port <port_number> --db <database_name> --username <username> --password <password> --out <database_output_file>
In the above command, we need to provide the hostname of MongoDB, port number on which it is running, the MongoDB database name, username & password for login, and the output filename.
The above command will backup on the specific database on your system. If you want to backup all databases on your MongoDB installation, you simply omit the –database argument and its value.
mongodump --host <hostname> --port <port_number> --db <database_name> --username <username> --password <password> --out <database_output_file>
In fact, if authentication is not enabled in your MongoDB server, then you can skip both –username and –password arguments.
Now that we know the basic command required to take a backup of MongoDB database, let us go about creating a shell script for it. Open terminal and run the following command to create an empty shell script mongo_backup.sh.
$ vi mongo_backup.sh
Add the following lines to store the different input parameters required for MongoDB backup. First we set the PATH variable (in case it is not set already) and also store today’s date in a variable. We will use it to name our backup file.
export PATH=/bin:/usr/bin:/usr/local/bin TODAY=`date +"%d%b%Y"`
Next, we add the following lines to set the database backup path, MongoDB host, port, username and password, and database name. Please replace these values as per your requirement.
DB_BACKUP_PATH='/backup/mongo' MONGO_HOST='localhost' MONGO_PORT='27017' MONGO_USER='test_user' MONGO_PASSWD='test_password' DATABASE_NAMES='test_db'
Next, we add the code to take backup of MongoDB database. First we create a folder with today’s date in folder name.
mkdir -p ${DB_BACKUP_PATH}/${TODAY}
Next, we have built the code to create backup in such as way that if you store DATABASE_NAME as ALL, then the script will take backup of all databases else it will loop through the databases mentioned in it one after the other in a space separated manner.
if [ ${DATABASE_NAMES} = "ALL" ]; then echo "You have choose to backup all databases" mongodump --host ${MONGO_HOST} --port ${MONGO_PORT} --username ${MONGO_USER} -password ${MONGO_PASSWD} --out ${DB_BACKUP_PATH}/${TODAY}/ else echo "Running backup for selected databases" for DB_NAME in ${DATABASE_NAMES} do mongodump --host ${MONGO_HOST} --port ${MONGO_PORT} --db ${DB_NAME} --username ${MONGO_USER} -password ${MONGO_PASSWD} --out ${DB_BACKUP_PATH}/${TODAY}/ done fi
In the above code, we have created backup of just 1 database test_db but if you want to get backup of multiple databases test_db1, test_db2, etc. just mention them one after the other in a space separated manner.
DATABASE_NAMES='test_db1 test_db2 test_db3'
If you want to take backup of all databases, set DATABASE_NAMES to ALL.
DATABASE_NAMES='ALL'
Please note, although MongoDB allows you to disable authentication while taking backup, we have not used it since it seems to be an insecure way of doing things.
Here is the complete code for reference.
export PATH=/bin:/usr/bin:/usr/local/bin TODAY=`date +"%d%b%Y"` DB_BACKUP_PATH='/backup/mongo' MONGO_HOST='localhost' MONGO_PORT='27017' MONGO_USER='test_user' MONGO_PASSWD='test_password' DATABASE_NAMES='test_db' mkdir -p ${DB_BACKUP_PATH}/${TODAY} if [ ${DATABASE_NAMES} = "ALL" ]; then echo "You have choose to backup all databases" mongodump --host ${MONGO_HOST} --port ${MONGO_PORT} --username ${MONGO_USER} -password ${MONGO_PASSWD} --out ${DB_BACKUP_PATH}/${TODAY}/ else echo "Running backup for selected databases" for DB_NAME in ${DATABASE_NAMES} do mongodump --host ${MONGO_HOST} --port ${MONGO_PORT} --db ${DB_NAME} --username ${MONGO_USER} -password ${MONGO_PASSWD} --out ${DB_BACKUP_PATH}/${TODAY}/ done fi
Make the script executable.
$ sudo chmod +x mongo_backup.sh
You can run the backup script as shown.
$ ./mongo_backup.sh
If you want to automatically run the above script everyday at 10.a.m then open crontab document.
$ crontab -e
Add the following line to it.
0 10 * * * /home/ubuntu/mongo_backup.sh
Save and close the file. Please note, you need to mention the full path to mongo_backup.sh shell script in the crontab file.
In this article, we have learnt how to create backup of MongoDB database. Depending on your requirement you can modify the above script to create backup or one or more, or even all databases on your system.
Also read:
How to Terminate Python Subprocess
How to Convert Epub to PDF in Linux
How to Convert Docx to PDF in Linux
How to Remove Yum Repository in Linux
How to Remove Repository in Ubuntu
Related posts:
How to Restore MongoDB Dump in Windows & Linux
VPS vs Shared Hosting : In-Depth Comparison
How To Clone Git Repository in Ubuntu
How to Change Element's Class in JavaScript
How to Remove Malware from Website
How to Redirect Using JavaScript
How to Embed PDF in HTML
How to Replace All Occurrences of String in JavaScript
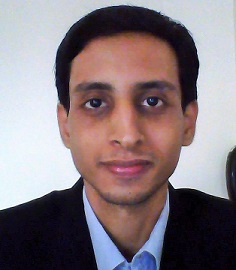
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.