Often you may need to redirect web pages on your websites. While it is recommended to redirect web pages & URLs from the server-side, sometimes you may really need to redirect pages from client-side using JavaScript. In this article, we will learn how to redirect using JavaScript.
How to Redirect Using JavaScript
Although most JavaScript libraries like Jquery provide readymade functions to redirect web pages, it is always easier and simpler to do it via plain JavaScript. There are the two ways to redirect using JavaScript. Let us say you want to redirect to www.example.com/new-home. In this case, add either of the following command to your web page’s JS code.
// similar behavior as an HTTP redirect window.location.replace("http://www.example.com/new-home"); // similar behavior as clicking on a link window.location.href = "http://www.example.com/new-home";
In the first case, the redirect will not create an entry in browser’s history, while the second case will create one, since it is like clicking on another URL from your page.
Please note
- You will not be able to redirect from HTTPS web page to an HTTP one, using JavaScript. Most browsers won’t allow you to do it. Using JavaScript, you can redirect from HTTP to HTTP, HTTP to HTTPS and HTTPS to HTTPS pages only. If you want to redirect from HTTPS to HTTP page, you will need to do it via server side.
- You can use full URL (e.g. http://www.example.com/new-home) or relative paths (e.g. /new-home). If you use relative path for redirection, the browser will redirect to the page, under same HTTP/HTTPS protocol without changing it. If you want to redirect from HTTP to HTTPS page, you need to specify the full URL
- Some web frameworks like Django require you to specify a trailing slash after URLs. If that is the case with your web framework, you need to specify the trailing backslash in the above statements. Otherwise, your framework will further redirect it to URL with trailing slash appended to it.
- If you add the redirection in the function handler for submit button, it may not work, since the browser will simply submit the form instead of redirecting the page. In such cases, you may submit form using AJAX and redirect based on button submission’s response.
Here are several other ways to redirect pages on browser. They produce the same result as the ones mentioned above.
// window.location window.location.replace('http://www.example.com/new-home') window.location.assign('http://www.example.com/new-home') window.location.href = 'http://www.example.com/new-home' document.location.href = '/new-home'
If you just want to go back to your previous page in your browser history, you can also use window.history to do this.
// window.history window.history.back() window.history.go(-1)
In this article, we have learnt how to redirect pages using JavaScript.
Also read:
How to Show All Users in MySQL
How to Upgrade All Python Packages using pip
How to Create Python Function with Optional Arguments
How to Import Python Module Given Full Path
How to Automate Backup in Python
Related posts:
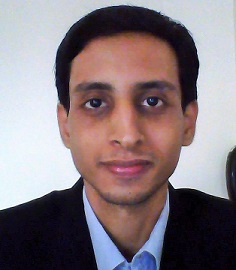
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.