These days almost every website and app requires software developers to send JSON data from client to server. Python developers too need to be able to send POST JSON data to web server and process server response. You can easily do this using Python Requests library. In this article, we have learnt how to POST JSON Data in Python requests.
What is Python Requests?
Python Requests is one of the most popular libraries, with more than 300 millions downloads. It allows you to easily work with HTTP requests – GET, POST, PUT, PATCH, etc. With this library, you do not need to encode your data or explicitly add query strings to URLs. Requests will do everything for you. Though there are tons of python libraries to interact with HTTP requests, none of them is as easy as this one. It also supports numerous session-related features such as Keep Alive, Connection Pooling, TLS/SSL verification, content compression & decompression, multi part uploads, connection timeouts and more. It seamlessly works with JSON data making it a popular choice for Python developers.
How to POST JSON Data in Python Requests
Let us look at how to send JSON data in Python requests.
1. Install Python Requests
By default, Python Requests is not present in Python and you will need to install it. Open terminal and run the following command to install it on your system
$ python -m pip install requests
2. Send POST JSON Data
You can easily send and accept requests in Python using requests library. It provides a function post() that allows you to include POST data in different formats. Let us say you want to send JSON Data to https://example.com/post from a python client to a web server.
Here is a simple code snippet to POST JSON Data in Python requests. You need to use this code in client side that is sending request to the server and displaying the status code of response returned by the server.
>>> import requests
>>> url = 'https://example.com/post'
>>> data = {"key": "value"}
>>> response = requests.post(url, json=data)
>>> response.status_code
200
First we import requests library. Then we define request URL and POST data. When we call post() function above, we need to pass two input parameters – request URL and post data. It returns response object that contains response code and data.
Please note, if your input data is in string format such as JSON string, then you need to use ‘data’ input parameter. If it is a Python dictionary then use ‘json’ input parameter. In second case, post() function will automatically convert it into JSON string.
## input data is string
>>> data = '{"key": "value"}'
>>> response = requests.post(url, data=data)
## input data is a dictionary
>>> data = {"key": "value"}
>>> response = requests.post(url, json=data)
You can check out the request payload using the following command.
>>> r.json()
Alternatively, you can also use json.dumps() to send data in JSON format. It basically converts python dictionary (and other objects) into their equivalent JSON format. Please remember to use json.dumps() and not json.dump().
In this case, we use data input parameter instead of json parameter.
import requests
url = "https://example.com/post"
data = {'key':'value'}
headers = {'Content-type': 'application/json', 'Accept': 'text/plain'}
response = requests.post(url, data=json.dumps(data), headers=headers)
In the above example, we have also supplied header information for request, using headers input parameter. This is required if you are sending data as a string. It tells the server that the string is a JSON string and not a regular string.
Sometimes your JSON data may be present in a file. In such cases, you need to extract JSON data from file before sending POST request.
3. Handle Response
The post() function will return a response object that you need to store for later use. It contains status_code attribute that contains response code. You can use its value to process further.
response = requests.post(url, json=data)
if response.status_code == 200:
print('Success!')
else:
print('An error occurred.')
4. Handle Errors
If there is an error in post() function’s inputs, then it will not return response object but throw an error. You can handle it by wrapping it in try…except block.
try:
response = requests.post(url, json=data, headers=headers)
response.raise_for_status()
except requests.exceptions.HTTPError as err:
print(err)
Conclusion
In this article, we have learnt how to POST JSON Data in Python. This is a common requirement in almost every website or app. There are many Python libraries that are available to handle HTTP requests. But Python Requests is one of the most popular ones among them. It allows you to easily send POST JSON Data to server, save and process its response. It also provides tons of other useful features. For more information about this library, check its official documentation.
Also read:
How to Remove SSL Certificate & SSH Passphrase in Linux
How to Capture Linux Signal in Python
How to Send Signal from Python
How to Clear Canvas for Redrawing in JS
How to Use Decimal Step for Range in Python
Related posts:
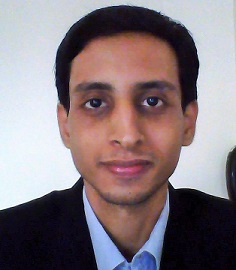
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.