JSON is a useful data type that allows you to store large amount of diverse data types in a compact manner. It is also easy to extract, search and retrieve data from JSON. Sometimes you may need to load JSON file in Python, import JSON file in Python or just read JSON file. In this article, we will look at how to extract data from JSON file in Python.
How to Extract data from JSON File in Python
Here are the steps to extract data from JSON file in Python. We will be using the json library which comes installed in Python, by default. It offers two functions json.load and json.loads to load JSON data in Python. We will look at which one to use when.
Let us say you have the following JSON file at /home/ubuntu/test.json
$ sudo vi /home/ubuntu/test.json
Add the following JSON to it.
[ { "Name": "Debian", "Version": "8", "Install": "apt", "Owner": "SPI", "Kernel": "4.8" }, { "Name": "Ubuntu", "Version": "18.10", "Install": "apt", "Owner": "Canonical", "Kernel": "4.11" } ]
Save and Close the file.
Also read : How to Install Laravel in NGINX
Load JSON File in Python
Before you read a JSON file, you will need to import JSON file in Python. Here is the command to do it. Let us say the above JSON file is located at /home/ubuntu/test.json
Here is the code to load JSON file in Python. We will use json.load function to load a JSON file
>>> import json >>> json_data = json.load('/home/ubuntu/test.json') >>> json_data[0].name >>> Debian
Also read : How to Check Cron Log in Linux
On the other hand, if you have json object then you need to use json.loads function. Here is an example.
>>> test_data = '{"a": 1, "b": 2, "c": 3, "d": 4, "e": 5}' >>> import json >>> json_data = json.loads(test_data) >>> print json_data >>> {u'a': 1, u'c': 3, u'b': 2, u'e': 5, u'd': 4} >>> print json_data['a'] >>> 1
That’s it. Use json.load() to import files and json.loads() to load strings in Python. As you can see it is quite easy to work with JSON files in Python.
Related posts:
How to Remove Duplicates from List in Python
How to Check if Directory Exists in Python
How to Copy List in Python
How to Store JSON to File in Python
How to Find Local IP Address in Python
How to Remove Trailing Newline in Python
How to Create PDF File in Python
How to Get Difference Between Two Lists in Python
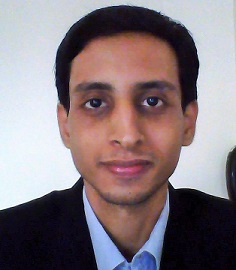
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.