Python allows you to easily work with files & directories. Sometimes you may need to create nested directory in Python. In this article, we will learn how to create nested directory in python. There are several ways to do this.
How to Create Nested Directory in Python
We will look at some of the different ways to create nested directory in Python.
1. Using mkdir
If you are using python >= 3.5, you can use pathlib.Path.mkdir function to create nested directory. For example, here is the command to create nested directory /home/data.
from pathlib import Path Path("/home/data").mkdir(parents=True, exist_ok=True)
In the above command, we call function Path and pass string to create a Path object. We further call mkdir() with parents=True so that it creates a nested directory. We also use exist_ok argument to create the directory even if it exists.
2. Using os.makedirs
You can also use os.makedirs() function to create nested directories.
import os if not os.path.exists('/home/data'): os.makedirs('/home/data')
os.makedirs allows you to directly make nested directories but the problem is that it throws an exception if the nested directory already exists. For this purpose, we call os.path.exists() to check if the folder exists or not.
In this approach, if the directory is created before it is checked it may give an error OSError. It is known as race condition, which may occur once in a while.
Alternatively, you can directly call makedirs() function within try catch block and catch the OSError whenever it occurs.
import os, errno try: os.makedirs('/home/data') except OSError as e: if e.errno != errno.EEXIST: raise
But using a blanket catch block for OSError does not completely solve the problem because it will fail to catch the error in case directory is not created due to insufficient permissions, full, disk, etc.
If you are using Python 3.3+, you can use the exist_ok=True argument to avoid this error. When you pass this argument, python will not give error, if the directory exists.
os.makedirs("/home/data", exist_ok=True) # succeeds even if directory exists.
In this article, we have learnt how to create nested directory in python using a couple of different ways. The main problem is that the creation of nested directory fails if it already exists, and gives an error. So it is advisable to first check if the nested directory exists, before you create it. If you are using python >=3.5 you can use either mkdir or makedirs to create the nested directory, since they both support the argument exist_ok to create directory even if it exists. With older python versions, it is better to first check if the nested directory exists before calling makedirs() or mkdir() functions.
Also read:
How to Add Blank Directory in Git Repository
How to Iterate Through Files in Directory in Python
How to Find All Text Files in Directory in Python
How to Convert CSV to Tab Delimited File in Python
How to Stress Test Linux Server
Related posts:
How to Sort List of Dictionaries by Value in Python
How to Create Cartesian Product of Two Lists in Python
How to Configure Python Flask to be Externally Visible
How to Read Inputs as Numbers in Python
How to Count Occurrence of List Item in Python
How to Check if Key Exists in Python Dictionary
How to Redirect Stdout & Stderr to File in Python
How to POST JSON Data in Python Requests
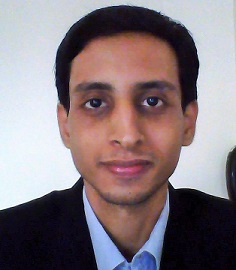
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.