Stdout is used by python to display text output of the commands that you run on your terminal or within a python script. Stderr is invoked when there is any error while running your command. This is also the case whenever you run any other command, not just python, and any other script such as shell script, in terminal. The problem is once these stdout and stderr messages are displayed they cannot be retrieved back later for future reference. The subsequent commands/scripts will push them out from display. In such cases, it is advisable to redirect stdout & stderr to file in Python. In this article, we will learn how to do this when you use python.
How to Redirect Stdout & Stderr to File in Python
In python, sys.stdout contains latest output of stdout and sys.stderr contains stderr output.
One of the ways to redirect these outputs to file is by setting them to a file object as shown below. Use this method if you want to redirect output from within script.
import sys with open('file', 'w') as sys.stdout: print('test')
Alternatively, you can redirect the output to a file(e.g. op.txt) using shell redirection.
$ python3 foo.py > op.txt
Similarly, you can also set stderr to file as shown below. If it doesn’t work for you, please try the next method mentioned below.
import sys with open('file', 'w') as sys.stderr: print('test')
If you are using python>3.4, you can use contextlib.redirect_stderr to redirect stderr outputs to file. Here is an example.
from contextlib import redirect_stderr with open('filename.log', 'w') as stderr, redirect_stderr(stderr): print(1/0) # errors from here are logged to the file.
In the above example, we have deliberately raised an exception by dividing 1 by 0. The error message is written to filename.log file.
Unlike redirecting stdout to file, it is a little tricky to send stderr output to file since the error occurrence generally breaks code execution. So it is advisable to use contextlib for such purposes.
In this article, we have learnt how to redirect stdout and stderr to file. You can use them capture output and error messages of your python commands and scripts for future reference.
Also read:
How to Extract Numbers from String in Python
How to Concatenate Items in List to String in Python
How to Create Multiline String in Python
How to Put Variable in String in Python
How to Fix ValueError: setting Array element in Sequence
Related posts:
Python Script to Check URL Status
How to Lock File in Python
How to Import Python Modules by String Name
How to Connect to PostgreSQL database using Python
How to Convert CSV to Tab Delimited File in Python
How to Find Django Install Location
How to Count Repeated Characters in String in Python
How to Get Difference Between Two Lists in Python
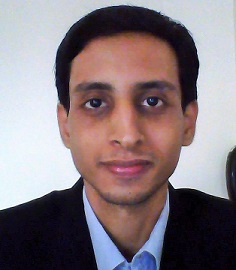
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.