Python allows you to perform many complex operations using concise lines of code and functions. Sometimes you may need to concatenate items in list into string in Python. Typically we need to loop through list items one by one and concatenate them to the result string. This can consume time & memory, especially if it is large list. In this article, we will learn how to do this easily using join() function, which is not only quicker but also consumes less memory.
How to Concatenate Items in List Into String in Python
Here are the steps to concatenate items in list into string in Python. Let us say you have the following list.
words = ['this', 'is', 'a', 'sentence']
Let us say you want to concatenate items in list into string, with spaces in between words.
this is a sentence
You can easily do this using the following command.
>>> ' '.join(words) 'this is a sentence'
If you want to use another character to join the list items, you can use that character or string, in place of ‘ ‘ above.
>>> '$'.join(words) 'this$is$a$sentence'
Please note, join function works only on string items. So if your list consists of non-string items such as [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] then you need to convert your list items into strings before calling join() function on them, as shown below. We have used map() function to quickly convert list items into strings, instead of looping through them.
>>> xs = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10] >>> ''.join(map(str, xs)) '12345678910'
join() function is faster than classical concatenation using for loop and concatenation operator ‘+’. This is because memory is allocated only once, in case of join(). In case of classical concatenation, memory is allocated separately every time you concatenate using ‘+’ operator.
In fact, it is fun to use join. It can even help you do useful tricks such as adding commas within your string, as well as add parentheses as shown below.
>>> ",".join("12345") '1,2,3,4,5' OR >>> ",".join("12345").join('()') '(1,2,3,4,5)'
In this article, we have learnt how to concatenate items in list in Python
Also read:
How to Put Variable in String in Python
How to Fix ValueError: setting array element with sequence
How to Fix ‘ASCII codec can’t encode’
How to Print Curly Braces in String in Python
How to Set Timeout on Function Call in Python
Related posts:
How to Sort CSV File in Python
How to Count Repeated Characters in String in Python
How to Get Difference of Two Dictionaries in Python
How to Convert Columns into Rows in Pandas
How to Drop One or More Columns in Python Pandas
Python: Reading & Writing to Same File
How to Get File Size in Python
How to Create Nested Directory in Python
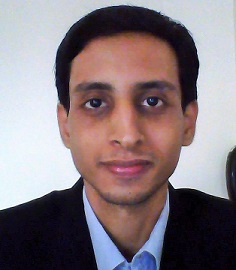
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.