Python dictionaries are powerful data structures that allow you to store diverse data types in one place. Sometimes you may need to get difference of two dictionaries in Python. In this article, we will look at how to calculate the difference between two python dictionaries.
How to Get Difference of Two Dictionaries in Python
Let us say you have the following two dictionaries
>>> dict1={1:'one',2:'two',3:'three'} >>> dict2={2:'two',3:'three'}
Here is the command to get the dict1’s keys that are not present in dict2.
Python 3.x
>>> diff = dict1.keys()-dict2.keys() set([1])
Python 2.x
>>> diff = dict1.viewkeys()-dict2.viewkeys() set([1])
Once you have the non-common keys you can rebuild the dictionary using their associated values, as shown below.
>>> new_dict={k:dict1[k] for k in diff} >>> new_dict {1: 'one'}
That’s it. As you can see it is quite easy to get difference between two dictionaries in Python. Depending on your python version, it is important to use the appropriate function to get the keys of your python dictionaries.
Also read : How to Intersect Python Dictionaries
Related posts:
How to Check Python Package Path
How to Execute Shell Command from Python
How to Convert CSV to Tab Delimited File in Python
How to Combine Multiple CSV Files Using Python
How to Import from Parent Folder in Python
How to get length of list in Django Template
How to Merge Folders & Directories in Python
How to Create PDF File in Python
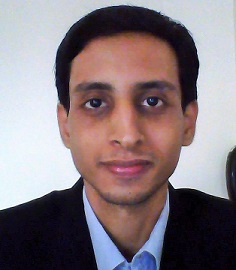
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.