Python is a powerful programming language that allows you to easily work with files and convert files from one format to another. Often we receive data as CSV files where data values are present in comma separated format. But we may need to convert them into tab delimited files to be able to use it in another software. This is especially true if you are doing data analysis using multiple software or platforms. In such cases, it is helpful to convert CSV to tab delimited file using Python. In this article, we will learn how to convert CSV files to tab delimited ones.
How to Convert CSV to Tab Delimited File in Python
Here are the steps to convert CSV to tab delimited file in Python.
First, create an empty python script file
$ vi csv_to_tab.py
Add the following lines to it to set its execution environment.
For Python 2 Files
#!/usr/bin/env python
For Python 3 Files
#!/usr/bin/env python
We will use python’s inbuilt library csv for converting CSV to tab delimited files. So add the following lines to import csv and os libraries.
import csv import os
Next, we store path to csv file in variable file by adding the following line. You can change the file path as per your requirement.
file='/home/ubuntu/data.csv'
Next, add the following lines which basically opens the csv file, reads the csv file line by line and writes its contents in a tab delimited format to the /home/ubuntu/data.txt file.
with open(file+'.csv','r') as csv_file: csv_reader = csv.reader(csv_file) csv_reader.next() ## skip one line (the first one) newfile = file + '.txt' for line in csv_reader: with open(newfile, 'a') as new_txt: #new file has .txt extn txt_writer = csv.writer(new_txt, delimiter = '\t') #writefile txt_writer.writerow(line) #write the lines to file`
Let us look at the above code. First, we use open() function to open csv file. We pass the file path to csv file to this function.
Next, we use csv.reader() to create a file reader for our file. It will help use traverse the file, line by line. Then we call next() function on the file to skip the first line, that is, the one with column headers. If you want to retain column headers in your tab delimited file, omit this line.
We define newfile variable to be the path to our tab delimited .txt file, which we obtain by adding .txt to csv file path.
Then we use open() function to open the text file. We run a for loop through the CSV file using csv_reader object. Each iteration will fetch the next line in CSV file. We use csv.writer() function to convert each line one by one to tab delimited format, by specifying delimiter option. Finally, we call writerow() function to write the content to file.
Save and close the file. Make it an executable with the following command.
$ chmod +x csv_to_tab.py
You can run the file with the following command.
$ python csv_to_tab.py
When you run the above code, you will find your tab delimited text file at /home/ubuntu/data.csv.txt. Here is the full code for your reference.
#!/usr/bin/env python import csv import os with open(file+'.csv','r') as csv_file: csv_reader = csv.reader(csv_file) csv_reader.next() ## skip one line (the first one) newfile = file + '.txt' for line in csv_reader: with open(newfile, 'a') as new_txt: #new file has .txt extn txt_writer = csv.writer(new_txt, delimiter = '\t') #writefile txt_writer.writerow(line) #write the lines to file`
If you want to convert multiple csv files located in a directory, modify the above code as shown below.
#!/usr/bin/env python import csv import os dir_path='/home/ubuntu' for file in os.listdir(dir_path): file_name, file_ext = os.path.splitext(file) if file_ext == '.csv': with open(file,'r') as csv_file: csv_reader = csv.reader(csv_file) csv_reader.next() ## skip one line (the first one) newfile = file + '.txt' for line in csv_reader: with open(newfile, 'a') as new_txt: #new file has .txt extn txt_writer = csv.writer(new_txt, delimiter = '\t') #writefile txt_writer.writerow(line) #write the lines to file`
In the above code, we store the directory path in dir_path variable. We use os.listdir() function to list all files and directories in this folder. We run a for loop through this list to run through each file and folder. In each iteration, we check if the file extension stored in file_ext variable is .csv. If so, we open the file and copy its contents to a corresponding tab delimited text file which we create by simply adding .txt extension to the csv file’s path. So if you have files data1.csv, data2.csv, data3.csv in the folder, their contents will be written to tab delimited files data1.txt, data2.txt, data3.txt.
In this article, we have learnt how to convert CSV file to tab delimited text file.
Also read:
How to Stress Text Linux Server
Schedule Cron Job Every 1 Hour in Linux
How to Send Message to Logged User in Linux
How to Delete User Accounts With Home Directory
How to Update or Change System Locale in Linux
Related posts:
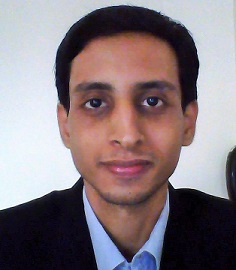
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.