Python lists allow you to store different types of data in one place and manipulate it easily. Sometimes you may need to concatenate or merge multiple lists in python. There are multiple ways to do this in python. In this article, we will look at the different ways to concatenate multiple lists in python.
How to Concatenate Multiple Lists in Python
Python provides tons of ways to concatenate lists. For our example, we will look at how to merge lists using
- itertools
- + operator
- * operator
- extend()
1. Using itertools.chain method
itertools.chain() method allows you to merge different iterables such as lists, tuples, strings, etc, and gives you a single list as output. It works on all data types in your iterables and is one of the fastest ways to merge lists.
Here is the syntax
itertools.chain(list1, list2, list3, ...)
Here is an example.
import itertools a = [1, 2, 3, 4] b = [5, 6, 7, 8] c = [9, 10, 11, 12] opt = list(itertools.chain(a,b,c)) opt [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12]
2. Using * operator
Please note, this operator is available only in python 3.6+. The * operator is a very efficient way to combine lists in python. If you prepend * to a list name it returns the index position of that list.
Here is the syntax
[*list1, *list2, *list3]
Here is an example.
a = [1, 2, 3, 4] b = [5, 6, 7, 8] c = [9, 10, 11, 12] opt= [*a, *b, *c] opt [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12]
In the above code, *a, *b, *c unwraps and presents the list items at the specified index of new list.
3. Using + operator
The + operator allows you to easily concatenate lists, just as you concatenate strings. Here is its syntax.
list1 + list2 + list3 + ...
Here is an example.
a = [1, 2, 3, 4] b = [5, 6, 7, 8] c = [9, 10, 11, 12] opt= a + b + c opt [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12]
4. Using Extend()
When you use extend() function, python performs in place extension of first list. But please note, this will append the items of second list into first list and modify it. If you want to concatenate lists without modifying them then don’t use this method.
a.extend(b) a [1, 2, 3, 4, 5, 6, 7, 8]
In this article, we have learnt how to merge two lists using various methods. If you want to combine multiple lists without modifying them use itertools, + or * operator. Out of them, * operator is available in python 3.6+. If you want to combine two lists and store the result in first one, then use extend() function.
Also read:
How to Get MD5 Hash of String in Python
How to Split String by Delimiter in Python
How to Read File Line by Line in Python
How to Recover Deleted Files in Linux
How to Use Key-Value Dictionary in Shell Script
Related posts:
How to Sort CSV File in Python
How to Compare Strings in Python
How to Convert Webpage into PDF using Python
Python Script to Check URL Status
How to Read File Line by Line Into Python List
Python: Reading & Writing to Same File
How to Flatten List of Lists in Python
How to Read Inputs as Numbers in Python
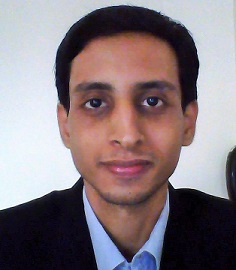
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.