Python is a popular programming language that offers many useful features. Sometimes you may need to check if URL is reachable, check server status, check if image URL is working and so on. Python makes it easy to quickly ping a URL and get its response code to determine if the URL is working or not. Here is python script to check URL status.
You can use this code to check if a website is working or not, if a link is broken, or if access is denied for URL.
Python Script to Check URL Status
Python provides many packages for this purpose. But we will see how to do this using 3 popular packages – urllib, requests and httplib.
1. Using urllib
urllib provides getcode() function to get response code of a URL. If the URL is up, the response will be 200.
Here is a simple code snippet to check if URL www.example.com is working.
import urllib.request url='http://www.example.com' status_code = urllib.request.urlopen(url).getcode() website_is_up = status_code == 200 print(website_is_up) #Output True
In the above example, please note, you need to specify the full URL along with protocol HTTP/HTTPS. Also getcode() function will return the response code of only the specified URL, not the entire website. If response code is 200, it only means that the specified URL is up and running. If the URL does not exist, it will throw URLError, and not return response code 404.
2. Using Requests
Requests is a python library available in python 2 & 3 and offers more features and flexibility over many other libraries, when it comes to processing requests.
In this case, we use requests.head() function to connect to the URL, and requests.response.status_code to get status code of given URL.
url = "https://www.example.com" request_response = requests.head(url) status_code = request_response.status_code website_is_up = status_code == 200 print(website_is_up) # OUTPUT True
Please note, requests.head() also does not check if a given URL exists or not and simply throws requests.exceptions.ConnectionError during connection with URL.
3. Using httplib
Like requests module, httplib first establishes a connection to a given URL using HTTPConnection() function, makes request using request() function, and gets response using getresponse() function. Here is an example to get response code of a given URL using httplib module.
import httplib conn = httplib.HTTPConnection("www.example.com") conn.request("HEAD", "/") r1 = conn.getresponse() print r1.status, r1.reason # output 200 OK
Unlike in urllib and requests module, you don’t need to mention the protocol in your URL. You can simply mention the www or non-www version of URL to check its status. Please note, httplib will return response code as 200, even if the server responds with 403 access forbidden response.
In this article, we have learnt how to check URL status in Python. You can use them to check if image URL is working or not, if a download file URL is still up & running. You can also use it in a loop to check if multiple URL status.
Also read:
How to Create Executable in Python
How to Store JSON Data in MySQL
How to Fix ‘Dpkg Was Interrupted’ Error in Linux
How to POST JSON Data in cURL
How to Store JSON Data to File in Python
Related posts:
How to Check if Variable is Number in Python
How to Download Images in Python
How to Store JSON to File in Python
How to Split Python List into N Sublists
How to Use Sleep Function in Python
How to List All Virtual Environments in Python
How to Remove Line from File Using Python
How to Sort CSV File in Python
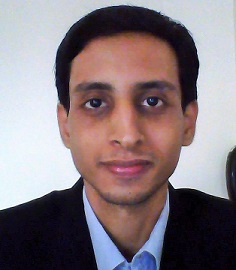
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.