Sometimes you may need to count repeated characters in string in Python. There are several ways to do this in Python. In this article, we will learn how to count repeated characters in string in Python. We will look at three ways to count repeated characters in python string – using dictionary, using list comprehensions and using collections.
How to Count Repeated Characters in String in Python
Here are the steps to count repeated characters in python string. Let us say you have a string called ‘hello world’.
1. Using dictionary
In this case, we initiate an empty dictionary. Then we loop through the characters of input string one by one. For each character we increment the count of key-value pair where key is the given character. If the given key doesn’t exist then we create a new key-value pair with key as the character and value as 1. So by the time you have looped through the entire string you have a dictionary of all the unique alphabets as keys and their counts as the values. Here is the code to do the same.
#!/usr/bin/env python input = "hello world" d = {} for c in input: try: d[c] += 1 except: d[c] = 1 for k in d.keys(): print "%s: %d" % (k, d[k])
2. Using Lists & Sets
You may also use List comprehensions to loop through the string and create a set of tuples where the first item is the character and the second item is its count. Here is an example to create a list of characters in string. In both the following cases, set function will convert the string into a list of its unique characters, including whitespace.
>>> s='hello world' >>> [(i,s.count(i)) for i in set(s)] [(' ', 1), ('e', 1), ('d', 1), ('h', 1), ('l', 3), ('o', 2), ('r', 1), ('w', 1)]
We can also do the same thing to create a dictionary of key-value pairs where the keys are the unique characters and values are their counts in input string.
>>> s='hello world' >>> {i:s.count(i) for i in set(s)} {' ': 1, 'e': 1, 'd': 1, 'h': 1, 'l': 3, 'o': 2, 'r': 1, 'w': 1}
3. Using Collections
Collections are dict subclasses but when you search for a key and it is not found, collections will create it for you, with value as 0. There are several types of collections available, most popular being defaultdict(int) and defaultdict(list) where you don’t need to write code for setting default values.
import collections input='hello world' d = collections.defaultdict(int) for c in input: d[c] += 1 for c in sorted(d, key=d.get, reverse=True): print '%s %6d' % (c, d[c])
In the above code, we create a collection ‘d’, all you need to do is simply loop through your input string and increment the dict values whose key is equal to the given character. Once you have looped through the entire string, you will have counts of all unique characters in string, including whitespaces.
In this article, we have learnt three different ways to count repeated characters in python string. You can use any of them as per your convenience. They all work well even with large strings. Generally, this code is a part of a larger function or module in a python application or website. So you can modify it as per your requirement.
Also read:
How to Write Limit Query in MongoDB
How to Copy/Transfer Data to Another Database in MySQL
How to Setup uwsgi with NGINX for Python
How to Check MySQL Version in Ubuntu
How to Clear Temp Files in Ubuntu
Related posts:
How to Remove All Occurrences of Value from List in Python
How to Configure Python Flask to be Externally Visible
How to Convert Columns into Rows in Pandas
How to Check if File Exists in Python
How to Redirect Stdout & Stderr to File in Python
How to Check if Substring is in List of Strings
How to Convert Bytes to String in Python
How to Copy List in Python
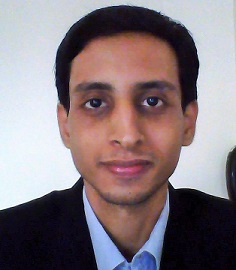
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.