Pandas is a popular module that helps you to import, export, transform data in Python. It offers tons of features to play with data, stored as tables. Often you may need to transpose columns into rows and vice versa, in your data. In this article, we will learn how to convert columns into rows in Pandas.
How to Convert Columns into Rows in Pandas
Let us say you have the following data in Python pandas dataframe df.
>>> print df location name Jan-2020 Feb-2020 March-2020 A "test" 12 20 30 B "foo" 18 20 25
Let us say you want to transform it into the following.
location name Date Value A "test" Jan-2020 12 A "test" Feb-2020 20 A "test" March-2020 30 B "foo" Jan-2020 18 B "foo" Feb-2020 20 B "foo" March-2020 25
To perform the above transformation, we use melt() function. Here is its syntax.
pandas.melt(frame, id_vars=None, value_vars=None, var_name=None, value_name='value', col_level=None, ignore_index=True)
In the above function, id_vars is the list of columns to be retained after transformation, var_name is the column to unpivot, and value_name is the value of the column to be used for unpivotting.
Here is the command to convert rows into columns, by unpivotting the separate date columns into a single column.
df.melt(id_vars=["location", "name"], var_name="Date", value_name="Value") location name Date Value 0 A "test" Jan-2010 12 1 B "foo" Jan-2010 18 2 A "test" Feb-2010 20 3 B "foo" Feb-2010 20 4 A "test" March-2010 30 5 B "foo" March-2010 25
When you run the above command what python pandas does is to pivot down all columns in input dataframe except the ones listed as id_vars, that is, location & name. While doing so, it will take all the remaining column names, create a new column called ‘Date’ and store these column names as Date column’s values. It will also take all the column values of the above mentioned column, create another column called Value and store all these values in it.
In this article, we have learnt how to convert columns into rows in Pandas.
Also read:
How to Set/Unset Cookie with jQuery
How to Abort AJAX Request in JavaScript/jQuery
How to Get Value of Text Input Field With JavaScript
How to Merge Two Arrays in JavaScript
How to Persist Variables Between Page Loads
Related posts:
How to Combine Querysets in Django
How to Sort CSV File in Python
How to Sort List of Dictionaries by Value in Python
How to Convert PDF to Text in Python
How to Check Version of Python Modules
How to Get MD5 Hash of String in Python
How to Create Python Dictionary from String
How to POST JSON Data in Python Requests
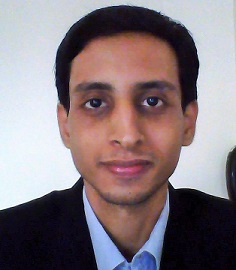
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.