Python provides many useful functions and features to manipulate strings present as variables or in files. Sometimes you may need to remove trailing newline in Python. In this article, we will learn how to do this.
How to Remove Trailing Newline in Python
You can easily remove trailing newline using rstrip() function. Here is its syntax.
string.rstrip([character_to_be_removed])
You can call rstrip() function on literal strings or string variables. If you don’t specify any option, it will remove all newline characters from your string. If you specify the character to be removed, it will remove only that character.
Let us say you have the following string with a trailing newline.
test = 'test string\n'
You can remove trailing newline from this string, with the following command.
>>> test.rstrip() 'test string'
Let us say you have the following string with different kinds of trailing whitespace characters.
test = 'test string \n \r\r\n\r \n\n'
You can remove all these spaces at one go, by just calling rstrip() function on your string.
>>> test.rstrip() 'test string'
But if you want to strip only newline characters then you can specify it in rstrip() function.
>>> test.rstrip('\n') 'test string \n \r\r\n\r '
rstrip() function is available in both Python 2 and 3.
In addition to rstrip(), python also supports strip() and lstrip() function. strip() function remove whitespace characters from both ends of the string, while lstrip() removes only leading whitespace characters. Here are some examples to illustrate their working.
>>> test = " \n\r\n \n abc \n\r\n \n " >>> test.strip() 'abc' >>> test.lstrip() 'abc \n\r\n \n '
In this article, we have learnt about different kinds of python functions to remove trailing as well as leading whitespaces.
Also read:
How to Pad String in Python
How to Get Size of Object in Python
How to Import from Parent Folder in Python
How to Attach Event to Dynamic Elements in JavaScript
How to Get Subset of JS Object Properties
Related posts:
What Is __name__ In Python
How to Stop Python Code After Certain Amount of Time
How to Create Cartesian Product of Two Lists in Python
How to Download Images in Python
How to Convert PDF to Text in Python
How to List All Virtual Environments in Python
How to Read File Line by Line Into Python List
How to Convert Columns into Rows in Pandas
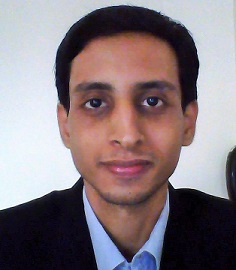
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.