JavaScript allows you to attach event handlers to DOM elements on your page. Generally, developers attach event handlers to static HTML elements such as buttons and links that are already defined on HTML page. If you try to attach an event handler to a dynamically created element then you will notice that this handler is not fired. For example, if you attach an onclick event to a dynamically generated link or button, it will not be fired. But in many cases, we do want such events to be fired. In this article, we will learn how to attach event to dynamic elements in JavaScript.
How to Attach Event to Dynamic Elements in JavaScript
You can attach event to dynamic elements using plain JavaScript as well as third-party libraries such as jQuery. Let us say you have a dynamic element with id=’myButton’. Let us say you want to call a function myFunction() when this button is clicked. Here are a couple of ways to do this.
1. Using JavaScript
Basically, you need to attach an event handler that is a parent or ancestor of your dynamic element, and is static in nature, that is, it is not dynamically created. Document, window, body, etc. are some of the elements that fulfil these 2 conditions. So here is an example where we attach an ‘click’ event to document object of the page.
document.addEventListener('click',function(e){ if(e.target && e.target.id== 'myButton'){ //do something } });
In the above code, we attach event listener to document. In the event handler, we check the ID attribute of the element that fired the event. If it is myButton, then we run the code that you want to be executed when the button with ID=myButton is clicked.
We use addEventListener() function which accepts the event type and handler function as arguments.
This works because whenever the button is clicked, the event handler attached to the clicking of its static ancestors is also triggered. So, every time you click the button, the click event for document is also triggered.
2. Using jQuery
You can also use jQuery library for the same purpose.
$(document).on('click','#myButton',function(){//do something})
This also works the same way. Instead of attaching event handler to dynamically generated button, you need to attach it to any of its ancestor which is statically created. So we attach the event handler to document object. We use on() function for this purpose. It allows you to specify the event type, target element, and handler function.
In this article, we have learnt how to attach event to dynamic elements in JavaScript. In all cases, you need to attach the event handler to a static ancestor of dynamic element. In the event handler, you need to check the ID of element that fired the event and process the event as per your requirement.
We need to follow this approach of the way JavaScript delegates events.
Also read:
How to get Subset of JS Object Properties
How to Close Current Tab in Browser Window
How to Get ID of Element That Fired Event in jQuery
How to Get Property Value of JS Objects
How to Scroll to Bottom of Div
Related posts:
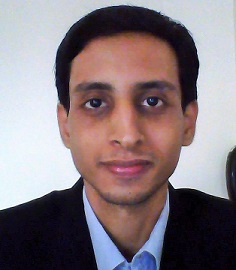
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.