Array is one of the most popular and powerful data types in JavaScript. It is used by almost every JS developer. Arrays offer tons of properties and functions. However, if you call them on variables that are not arrays then you may end up getting an error and your code execution might stop. So it is advisable to check if a variable is array before calling any array-specific functions or properties on it.
How to Check if Variable is Array in JavaScript
There are several simple ways to check if a variable is array in JavaScript.
1. Using constructor
Every JS variable has constructor property readily available, which contains its data type. You can check if variable is array or not as shown below. It returns true if the variable is array, else it returns false.
variable.constructor === Array
If your variable is test_data then here is the command to check if it is array or not.
test_data === Array
You can also use it to test if a JS object property is array or not. For example, let us say you have the following JS object.
test_data={'a':[1,2,3],'b':1}
You can use the above method on JS object property as shown below.
test_data.a === Array // returns true test_data.b === Array // returns false
The above method is the fastest way to check if a variable is array or not, on all modern browsers such as Chrome, Firefox, Edge, Safari, etc. You can be extra cautious and check if the constructor property exists in the first place.
variable.prop && variable.prop.constructor === Array
2. Using isArray
The Array object is available by default in most modern browsers (Chrome, Firefox, Safari, Edge). You can also use the Array object’s isArray function, to check if a variable is array or not.
Array.isArray(variable)
You can also call this function object’s property, not just simple variables. Here is an example to call the function test_data.a property defined above.
test_data={'a':[1,2,3],'b':1} Array.isArray(test_data.a) //returns true b=1 Array.isArray(b) //returns false
3. Using Object API
You can also use the Object API available in every modern web browser to get the object type of your variable, as shown below.
Object.prototype.toString.call(variable) === '[object Array]';
This is method is slower than the others but it allows you to check variable against any data type, not just Array. All you need to do is change [object Array] to the data type string of your requirement.
This method can also be used on simple variables as well as JS object properties.
a = [1, 2, 3]; b = 1; test_data={'a':[1,2,3],'b':1} Object.prototype.toString.call(a) === '[object Array]' // returns true Object.prototype.toString.call(b) === '[object Array]' // returns false Object.prototype.toString.call(test_data.a) === '[object Array]' // returns true
In this article, we have described 3 simple ways to check if variable is array or not. If you want the fastest way to do this, go for the first method. If you want the most flexible way to do this, go for the 3rd method.
Also read:
How to Get Highlighted/Selected Text in JavaScript
How to Call JS Function in Iframe from Parent Page
How to Prevent Page Refresh on Form Submit
How to Check if JS Object is Array
Regular Expression to Match URL Path
Related posts:
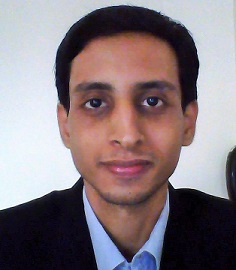
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.