JavaScript arrays are powerful data structures used by almost every web developer. It provides tons of features and functions. Sometimes you may need to remove empty element from JS array. This is often required if you have received data from another source and need to clean it up before using it. There are several ways to do this. In this article, we will learn how to remove empty element from JS array.
How to Remove Empty Element from JS Array
Let us say you have the following JS array.
var arr = [1,2,,3,,-1,null,,0,,undefined,5,,6,,7,,8,,,,];
We will look at some of the simple ways to quickly remove empty elements from the above array. By empty elements we mean null, undefined and blanks.
1. Using filter
Filter functions allow you to loop through an array, perform tasks for each array, and return an array of elements that fulfill that task or condition specified in filter. Here are 3 ways to use filter functions to remove empty elements from your array.
arr.filter(n => n) // [1, 2, 3, -1, 5, 6, 7, 8] arr.filter(Number) // [1, 2, 3, -1, 5, 6, 7, 8] arr.filter(Boolean) // [1, 2, 3, -1, 5, 6, 7, 8]
In the first filter function, we just check if the number is greater than or equal to itself. It returns all array elements that fulfill this condition. Nulls, undefined value and blank elements do not fulfill this condition so are not present in result array.
The second filter checks if each array element is a Number, using Number() function, while the 3rd filter checks if each element evaluates to proper Boolean. In case of null, undefined and blanks, they will return false when passed to Boolean() function.
If you want to remove only nulls and undefined but retain others then you can modify your filter callback function as shown below.
var filtered = arr.filter(function (el) { return el != null; });
In this case it returns elements that are not null or undefined.
2. Using Join & Split
You can also use a combination of Join and Split functions to remove empty elements from array, provided they are all text or string elements.
Let us say you have the following array.
var arr = ['1','2',,'3',,'-1',null,,'0',,undefined,'5',,'6',,'7',,'8',,,,];
You can use join() and split() function to remove empty elements as shown below.
arr.join('').split(''); // ['1', '2', '3', '-1', '5', '6', '7', '8']
The join() function concatenates all the strings into a single text, omitting nulls, undefined and blanks in the process. The split() function splits each character of the text into a separate array element.
In this article, we have learnt how to remove empty element from JS array. Using filter() is one of the simplest and most flexible ways to do this. You can customize filter callback functions as per your requirement.
Also read:
How to Check if Variable is Array in JavaScript
How to Get Highlighted/Selected Text in JavaScript
How to Call JS Function in Iframe from Parent Page
How to Prevent Page Refresh on Form Submit
How to Check if Object is Array in JavaScript
Related posts:
How to Get Highlighted/Selected Text in JavaScript
How to Allow only Alphabet Input in HTML Text Input
How to Generate Random Color in JavaScript
How to Split Array Into Chunks in JavaScript
How to Auto Resize IFrame Based on Content
How to Get Unique Values from Array in JavaScript
How to Get URL Parameters Using jQuery or JavaScript
How to Check if Variable is Array in JavaScript
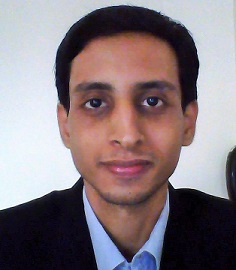
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.