We often pass URL parameters in GET requests on our websites and applications. These parameters are typically used by client side as well as server side to customize web pages. Sometimes you may need to read or get URL parameters to analyze them further. In this article, we will learn how to get URL parameters using JavaScript.
How to Get URL Parameters Using jQuery or JavaScript
Let us say you have the following URL.
http://example.com/?technology=jquery&blog=testblog
Let us say you want to obtain URL parameters & their value, for technology (jquery) and blog (testblog).
There are several ways to do this. We will look at a couple of simple ways that does not require third-party JS libraries.
1. Using URLSearchParams
Most modern browsers such as Chrome, Firefox, Safari and Edge support URLSearchParams() function that returns all the URL parameters as a JS object of key-value pairs. Each key is the parameter name and each property value is the parameter value. Here is an example to retrieve URL parameters from present URL in browser. We use window.location.search to obtain query string.
var url_params = new URLSearchParams(window.location.search);
Now url_params will contain all URL parameters as key-value pairs.
url_params = {'technology':'jquery','blog':'testblog'}
If you want to check for a particular URL parameter you can do so using has() function, as shown below. It returns true if the key is present else it returns false.
url_params.has('technology');//returns true url_params.has('server');//returns false
If you want to retrieve a particular URL parameter value, you can do it with get() function.
param = url_params.get('technology'); console.log(param); //returns jquery
2. Manual Splitting of URL string
If you are using an old browser that does not support URLSearchParams() function, then you will need to manually split the string into individual parameters first, and then split each parameter into property and value, as shown below.
var url_string = window.location.search.substring(1), url_params = url_string.split('&'), param, i; for (i = 0; i < url_params.length; i++) { param = url_params[i].split('='); }
In the above code, we use window.location.search to obtain URL string of current URL in browser. We call substring() function to remove the first character, which is ‘?’, from this string.
Next, we call split() function on this string to split it into individual parameters. We split the URL string by ‘&’ character. For example, this will split URL string ‘technology=jquery&blog=testblog‘ into ‘technology=jquery’ and ‘blog=testblog’
All the split strings are stored in an array named url_params. We loop through this array, and further split individual parameter string by ‘=’ character, and store the result in param array. param[0] contains the parameter name (e.g. technology) and param[1] contains the parameter value (e.g. jquery).
Now you can use them as per your requirement.
In this article, we have learnt a couple of easy ways to parse URL string and get URL parameter using JavaScript. We recommend using the first method since it readily gives URL parameters with value in a neat JS object.
Also read:
How to Convert String to Date in MySQL
How to Calculate Age from DOB in MySQL
How to Escape HTML Strings with jQuery
How to Check if Element is Visible in jQuery
Set NGINX to Catch All Unhandled Virtual Hosts
Related posts:
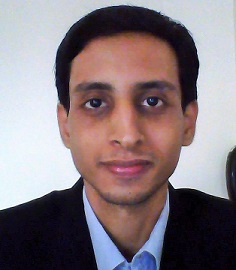
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.