JavaScript is a powerful language that allows you to perform many useful DOM operations on your web pages. But sometimes you may need to generate random string characters in JavaScript. This is often required if you want to generate random key or password for your user. In this article, we will learn how to generate random string characters in JavaScript.
How to Generate Random String Characters in JavaScript
Here are the steps to generate random string characters in JavaScript. First we will define our character set to be used for generating our string. If you want to include a-zA-Z0-9 then here is a character list.
var characters = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789';
You can also include special characters in the string, as per your requirement. On the other hand, if you only want numbers and not alphabets, then you can modify the above string as shown.
var characters = '0123456789';
Next, if you need a random string of n characters, we will run a loop with n iterations, and in each iteration, we will pick a random letter from the above character set. For this purpose, we need to calculate a random index in each iteration. For this, we will use random() function. random() function returns a floating point number between 0 and 1, excluding 1. We will multiply this random number with the total length of our character set, and round it to nearest integer, to obtain a random index. We will use this random index to pick a random letter from our string.
Putting it together,
function generate_string(length) { var result = ''; var characters = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789'; var charLength = characters.length; for ( var i = 0; i < length; i++ ) { result += characters.charAt(Math.floor(Math.random() * charLength)); } return result; }
In the above function, we accept length of random string to be generated as input. We create result variable to store result string, and assign it as empty string. Our character set is already defined above. We store length of character set in charLength variable. We run for loop length number of times. In each iteration, we calculate the random index using the formula Math.random() * charLength and round it to the nearest integer with Math.floor() function. We use this index to pick a random letter using characters.CharAt() function. In each iteration, we append the randomly picked letter to the result string.
When the loop completes, we have out random string generated.
You can call it as shown below. Here is an example to generate string of 5 characters.
console.log(generate_string(5));
If you want to insert certain characters such as hyphen at specific positions (e.g. abcd-srfe-eree-rerer), you can do so by modifying the above for loop. Here is an example to insert ‘-‘ after every 4 characters.
function generate_string(length) { var result = ''; var characters = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789'; var charLength = characters.length; for ( var i = 0; i < length; i++ ) { if(i%5!=0){ result += characters.charAt(Math.floor(Math.random() * charLength)); }else{ result+='-'; } } return result; }
In this article, we have learnt how to generate random string using JavaScript. This is very useful to generate keys and passwords in your web pages. You can also send it to your backend server to be saved into your database, for later use.
Also read:
How to Fix PostgreSQL Error Fatal Role Does Not Exist
How to Install Python Package Using Script
How to Iterate Over List in Chunks
How to Create Python Dictionary from String
How to Strip HTML from Text in JavaScript
Related posts:
How to Disable/Enable Input in jQuery
How to Deep Clone Objects in JavaScript
How to Remove All Child Elements of DOM Node in JS
How to Check if Variable is Array in JavaScript
How to Get URL Parameters Using jQuery or JavaScript
How to Replace Line Breaks With <br> in JavaScript
How to Add Days to Date in JavaScript
How to Return Response from Asynchronous Call in JavaScript
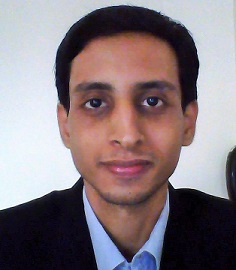
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.