Websites and applications often contain user input elements such as textbox and textarea. Sometimes these user inputs may contain line breaks. But line breaks cannot be used to create new line in HTML. This may require you to modify input text before you save it to your database. For example, you may receive a plain string with line breaks and may need to replace them with <br> tags, if you want to display it as HTML. This is also true if you already have data containing line breaks and want to replace them with <br> tag. In this article, we will learn how to replace line breaks with <br> in JavaScript.
Why Replace Line Breaks with <br> in JavaScript
Line breaks cannot be displayed on HTML pages to create a newline. In HTML, you need to use <br> for line break. If user input contains line breaks then you need to replace it with <br> tag. Otherwise, these line breaks will be stored in your database and rendered as it is on your web pages, without creating a new line. It is advisable to replace line breaks using JavaScript, on client web browser, before sending the data to server. This will save a lot of unnecessary processing on server side.
How to Replace Line Breaks With <Br> in JavaScript
There are a couple of simple ways to replace line breaks with <br> in JavaScript. We will learn each of these methods one by one.
1. Using Replace() Function
You can easily do this using replace() function. Here is the syntax of replace() function
string.replace(old_substring, new_substring);
In the above function, we need to provide old substring or regular expression for the substring, that you want to replace, as first argument, and the new substring as the second argument. If you use just a plain substring as first argument, replace() function will replace only the first occurrence of the substring. If you want to replace all occurrences of substring, you need to use a regular expression for the substring to be replaced.
Let us say you have the following plain string with line breaks, as shown below.
var str = "Hello World. Good Morning."
Let us say you want to replace the above string with the following.
"Hello World.<br/> Good Morning."
You can easily do this using the following command.
str = str.replace(/(?:\r\n|\r|\n)/g, '<br>'); OR str = str.replace(/(?:\r\n|\r|\n)/g, '<br/>');
In the above code, the first argument of replace() function is a regular expression to match all types of line breaks, irrespective of OS (Linux, Windows, Mac). Some OS uses \n as line breaks, some use \r\n and some use \r. This regular expression will replace all of those. If you want to replace only some of them like \n but not \r then you can customize the above expression as per your requirement. Also, we have used ?: at the beginning of regular expression to specify a non-capturing group of regex, that is, it will not be saved in memory for future reference, making it more efficient. You can omit it if you want to.
2. Using Split-Join
In this solution, we do not need a regular expression. We split the input string using split() function, with lie break character as the delimiter, instead of splitting text based on whitespace characters. This will give us an array of substrings. We can use join() function to put the string back together, with <br> character as the delimiter. This time, the line break character will be replaced by <br> tag.
var str = "Hello World.
Good Morning."
str = str.split('\n').join('<br/>');
The split(‘\n’) function splits string into array of substrings by splitting original string based on line break. Join(‘<br/>’) method joins the array by adding <br/> between the array elements.
In this article, we have learnt how to replace line breaks with <br> tag in JavaScript.
Conclusion
In this article, we have learnt how to replace line break characters with <br/> tag. You can use either of the two solutions described above. The first solution is a little complicated due to the presence of regular expression but is flexible enough to handle complicated cases. The second method is simple and easy to understand. But it is important to replace line breaks with br tag on client side, using JavaScript or some other JS library. Otherwise, once it is passed to the server and stored in database, it becomes a headache to modify the data while rendering HTML page.
Also read:
How to Detect When User Leaves Web Page
How to Generate PDF from HTML Using JavaScript
How to Auto Resize IFrame Based on Content
How to Count Substring Occurrence in String in JS
How to Convert Comma Separated String into JS Array
Related posts:
How to Get URL Parameters Using jQuery or JavaScript
How to Format Number as Currency String
How to Detect When User Leaves Page
How to Remove Empty Element from JS Array
How to Persist Variables Between Page Load
How to Attach Event to Dynamic Elements in JavaScript
How to Return Response from Asynchronous Call in JavaScript
How to Change Element's Class in JavaScript
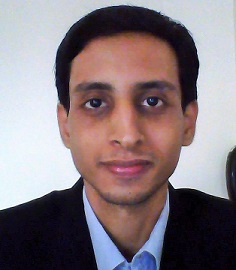
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.