Often we need to display information as currency in websites and apps. In most cases, these values are stored as plain integers, floats or decimal point numbers in our back end systems. In such cases, we need to format numbers as currencies before displaying them. In this article, we will learn how to format number as currency string using JavaScript. There are several ways to do this and we will look at a couple of simple methods.
How to Format Number as Currency String
Here are some of the ways to format numbers are currencies.
1. Using Intl.NumberFormat
As part of internationalization API, JavaScript already provides a number formatter. Here is a sample code to format value 2500 as $2,500.
// Create our number formatter. var formatter = new Intl.NumberFormat('en-US', { style: 'currency', currency: 'USD', }); formatter.format(2500); /* $2,500.00 */
The formatter takes 2 arguments – currency code such as en-US and JS object with additional options such as style & currency. Here are a couple of additional options to round numbers to nearest decimal place.
minimumFractionDigits: 0, // (sufficient for whole numbers, but prints 2500.10 as $2,500.1) maximumFractionDigits: 0, // (causes 2500.99 to be printed as $2,501)
In the above example, we have specifically formatted number as dollars. Here is a list of currency codes you can use to format numbers as various currencies. But if you want to format it as per client system’s locale, then use undefined in place of ‘en-US’.
Please note, the above code will only add currency symbol such as $ and comma separators in your number. It will not perform currency conversion if you change currency code.
2. Using toLocaleString
toLocaleString() is another function with a similar syntax, to convert number to currency. Here is an example to convert 2500 to $2,500 using toLocaleString().
(2500).toLocaleString('en-US', { style: 'currency', currency: 'USD', }); /* $2,500.00 */
toLocaleString() is a much older function than Intl.NumberFormat but it is a lot slower if you need to convert many numbers into currencies.
So if your client uses an old browser or IE, then you may want to use toLocaleString(). On the other hand, if you want to display many numbers as currencies, then it is advisable to use Intl.NumberFormat.
One Note
Please note, some European nations use commas in place of decimals and dots in place of commas. In such cases, you need to replace commas with dots and vice versa in your currency, using replace() function. Here is a simple example to illustrate this.
var x = formatter.format(2500); /* x = $2,500.00 */ var parts = x.toString().split("."); //split number into 2 parts - before and after decimal parts[0] = parts[0].replace(/,/g, "."); //replace comma with dot parts[1] = parts[1].replace(/,/g, "."); //replace comma with dot x = parts[0] + ',' + parts[1]; //join 2 parts back console.log(x) //$2.500,00
In this article, we have learnt how to format number as currency string. You can also use it to format numbers as different currencies such as Euro, Yen, etc.
Also read:
How to Access iFrame Content With JavaScript
How to Convert Form Data to JSON in JavaScript
How to Check if Element is Visible After Scrolling
How to Use Variable in Regex in JavaScript
How to Use Variable in Regex in Python
Related posts:
How to Detect Tab/Browser Closing in JavaScript
Remove Accents/Diatrics from String in JavaScript
How to Detect Invalid Date in JavaScript
How to Store Data in Local Storage in JavaScript
How to Generate Random Color in JavaScript
How to Get Property Values of JS Objects
How to Match Exact String in JavaScript
How to Get Value of Text Input Field With JavaScript
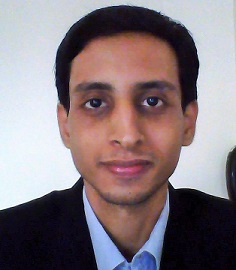
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.