JavaScript is often used to process form elements on web pages. Text input field is a commonly used form element, and it can also be used outside forms, in a standalone way. Often you will need to be able to read text input field’s value to process it further. In this article, we will learn how to get value of text input field with JavaScript. There are several ways to do this.
How to Get Value of Text Input Field With JavaScript
Let us say you have the following text input field.
<input name="searchBox" type="text" id="searchTxt" class="searchField"/>
There are several ways to get value of text input field with JavaScript.
1. Using ID
You can easily get value of text input field using its id attribute. Here is an example to get input field value using its ID. Replace the part in bold below with the ID of your input text.
var input_value = document.getElementById("searchTxt").value;
2. Using Class Name
You can also use the class name to identify the text input field. Here is syntax to get text input field value using its class name.
document.getElementsByClassName('class_name')[whole_number].value
Here is an example of it. Replace searchField with the class name of your text input field.
var input_value = document.getElementsByClassName("searchField")[0].value;
Also you need to replace 0 above with the index of your input. This is because getElementsByClassName returns a collection of all DOM elements on your page, with matching class name. So you need to specify the exact index of your target element. In our example, we need the first element with matching class so we use 0 index value.
3. Using Tag Name
You can also use tag name to identify the element on your page, with the help of getElementsByTagName() function. Here is the syntax of that command.
document.getElementsByTagName('tag_name')[whole_number].value
Here is an example to get input field.
document.getElementsByTagName("input")[0].value;
Here also we need to specify the index number (e.g. 0) to get the first element with matching tag name (e.g. input). This is because getElementsByTagName will return a collection of all DOM elements on your page that match the given tag name.
4. Using Name Attribute
You can get input field value by selecting via its name attribute. Here is the syntax of such a command.
document.getElementsByName('name')[whole_number].value
Here is an example to get value of 1st text input field with name=searchTxt.
document.getElementsByName("searchTxt")[0].value;
getElementsByName() function returns a collection of elements whose name matches given value. So we need to specify the index number of the element. We have specified 0 above to get the 1st matching element whose name is searchTxt.
In this article, we have learnt how to get text input field value. Methods 2, 3, 4 require you to specify an index because there can be multiple elements with same class, tag or name attributes on a singe page, and you need to specify the exact element whose value you want.
Also read:
How to Merge Two Arrays in JavaScript
How to Persist Variables Between Page Loads
How to Scroll to Element with JavaScript
How to Scroll to Element with jQuery
How to Add Delay in JavaScript Loop
Related posts:
How to Access Iframe Content With JavaScript
How to Get Element's Outer HTML using jQuery
How to Find Sum of Array of Numbers in JavaScript
How to Detect Invalid Date in JavaScript
How to Check if Object is Array in JavaScript
How to Get Highlighted/Selected Text in JavaScript
How to Display Local Storage Data in JavaScript
How to Get Position of Element in HTML
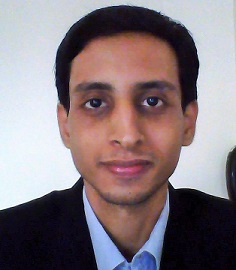
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.