JavaScript loops are commonly used to perform iterative tasks in websites and applications. But by default, each iteration of JS loop runs immediately after the previous one, without any pause or delay. But sometimes you may want to add delay/sleep in a JS loop, whereby the JS execution thread waits for a while after previous iteration.
How to Add Delay in JavaScript Loop
Typically developers use setTimeout() function to add delay/sleep in their code. This function waits for a specific amount of time before executing a given set of code or function. Here is an example that developers use to add delay of 1 second in each iteration of for loop.
for(var start = 1; start < 10; start++) { setTimeout(function () { alert('hello'); }, 1000); }
But the above code will not delay each iteration by 1 second. This is because setTimeout function is non-blocking and returns control immediately. So only the first alert will be displayed after a delay of 1 second but the rest are displayed immediately one after the other, without any delay.
Instead you need to modify your function such that each iteration is called after specified delay of 3 seconds.
var i = 1; // set your counter to 1 function myfunc() { // create a loop function setTimeout(function() { // call a 3s setTimeout when the loop is called console.log('hello'); // your code i++; // increment the counter if (i < 10) { // if the counter < 10, call the loop function myfunc(); // .. again which will trigger another } // .. setTimeout() }, 3000) } myfunc();
Let us look at the above code in detail. First we set a global counter to 1. In our function myfunc() we call setTimeout(), with a time out value of 3 seconds. So it will execute the code present as its 1st argument, only after 3 seconds. This code’s 1st line prints ‘hello’ in console. Then it increments the global counter value and checks if it is less than 10. If so, it calls myfunc() function again. When this function is called the same cycle repeats, and again the setTimeout() function in the called myfunc() function will execute only after 3 seconds. If the global counter value is equal to 10, then it does not do anything.
This repeats 10 times until myfunc() is not called anymore.
In the above code, replace the part which says ‘your code’ with the code that you want to execute in each iteration after adding required delay/sleep.
In this article, we have learnt how to add delay in JavaScript loop.
Also read:
How to Use Dynamic Variable Names in JavaScript
How to Get Difference Between Two Arrays in JavaScript
How to Compare Dictionary in Python
How to Compare Objects in JavaScript
How to Group by Array of Objects by Key
Related posts:
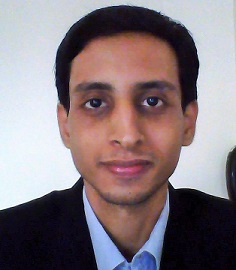
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.