JavaScript Objects are great way to store & retrieve data in key-property format. They allow you to store variables, arrays, dates, functions and even references in place. Sometimes you may need to delete specific key from JS object. In this article, we will learn how to remove key from JavaScript Object.
How to Remove Key from JavaScript Object
You can easily delete key from JavaScript Object using delete statement. Here is the syntax for it.
delete object.keyname; or delete object["keyname"];
Let us say you have the following object in JavaScript.
var thisIsObject= { 'Cow' : 'Moo', 'Cat' : 'Meow', 'Dog' : 'Bark' };
Let us say you want to remove the key ‘Cow’ from your JS object.
Here are the different commands to do this. They all result in deletion of specified key as well as its property value. The only way to remove a key from JS object is to remove its property using delete command.
// Example 1 var key = "Cow"; delete thisIsObject[key]; // Example 2 delete thisIsObject["Cow"]; // Example 3 delete thisIsObject.Cow;
In the first example, we assign the key to be deleted to a variable pass it to the object name to retrieve its property. We use delete command to delete it. This is useful if your key names are present in variables. It is also useful if you want to loop through the elements of object and delete multiple keys one after the other.
In second example, we pass the literal key value to retrieve the property and in third example we use the dot notation to access and delete its property.
In this article, we have learnt several different ways to delete key from JS object. You can use any of them as per your requirement.
Also read:
How to Deep Clone Objects in JavaScript
How to Include JavaScript File In Another JavaScript File
How to Add Event to Dynamic Elements in JavaScript
How to Clone Objects in JavaScript
How to Replace All Occurrences of String in JavaScript
Related posts:
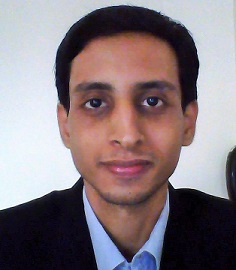
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.