Sometimes you may need to get client IP address from web browser using JavaScript. Typically, this information is retrieved by web server while serving request. In this article, we will learn how to get this information. You may need to use a third-party web service for this purpose.
How to Get Client IP Address Using JavaScript
There are a couple of ways to get client IP address in JavaScript.
1. Using Third Party Services
There are many third-party services that offer IP lookup. They basically have the same setup. You need to obtain an API key from their website, after registering with them. Then you need to send a request from the browser to the service’s API URL to obtain the IP address of browser.
First include the path to the jQuery library in your web page. You can also do this via JavaScript but jQuery makes it super easy.
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/2.2.4/jquery.min.js"></script>
Then add the following lines to your web page’s script tag. Replace your API key below. We have used BigDataCloud service for IP loopkup.
// Base let apiKey = '<your_api_key>'; $.getJSON('https://api.bigdatacloud.net/data/ip-geolocation?key=' + apiKey, function(data) { console.log(JSON.stringify(data, null, 2)); });
You can also use Abstract API.
$.getJSON('https://ipgeolocation.abstractapi.com/v1/?api_key=' + apiKey, function(data) { console.log(JSON.stringify(data, null, 2)); });
There are many such services available for IP lookup. Basically, you need to get your API key and insert it into the URL for IP lookup as per their specification.
This approach is useful if your web page is created using third-party services like SquareSpace that do not offer much room to customize your back end functions. If your website is running on your own server then just follow the method described below.
2. Using Own Server
In this case, you just need to send a dummy request to your own web server, which will be able to easily retrieve the IP address of your client browser and return the response as JSON or text. You may have realized that the above third-party services also do the same thing. When you send a request to their API, it is received by their web server, which in turn, extracts the IP address of the client browser and returns the information back to the browser. Replace the URL in bold with your website URL that returns IP address of request.
$.getJSON('https://example.com/get_ip', function(data) { console.log(JSON.stringify(data, null, 2)); //do your stuff });
In this article, we have learnt how to get client IP address using JavaScript. It is useful if you want to customize your website, depending on the IP address range, or you want to block/allow your website content for specific IP addresses. In both the above approaches, you need to send the request to a server from your web browser, to get IP address.
Also read:
How to Break ForEach Loop in JavaScript
How to Validate Decimal Number in JavaScript
How to Fix Uncaught ReferenceError in jQuery
How to Detect Browser in JavaScript
How to Get Array Intersection in JavaScript
Related posts:
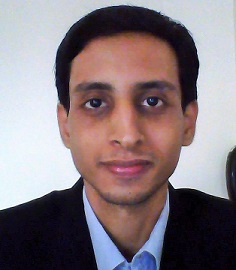
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.