While working with web development, there may be situations where we need to parse a string/number and verify if it is a decimal number or not. In this article, we will learn how to validate decimal number in JavaScript.
How to Validate Decimal Number in JavaScript
By default, most developers use isNumeric() function to determine if a string/number is decimal number or not.
isNumeric(n)
But isNumeric() function fails in many use cases as shown below.
// Whitespace strings: IsNumeric(' ') == true; IsNumeric('\t\t') == true; IsNumeric('\n\r') == true; // Number literals: IsNumeric(-1) == false; IsNumeric(0) == false; IsNumeric(1.3) == false; IsNumeric(9e5) == false;
So we need to make use of two JS functions for this purpose isNaN(), parseFloat() and isFinite(). parseFloat() parses an argument and returns a floating point number. isNaN() checks if a given value is NAN (Not a Number), which can be the result of parseFloat() in case the input is not a number. We also use isFinite() function to determine if it is not infinite. Combining these you can check if a number n is decimal or not as shown below.
return !isNaN(parseFloat(n)) && isFinite(n);
You can create your own isNumeric() function incorporating the above expression.
function isNumeric(n) { return !isNaN(parseFloat(n)) && isFinite(n); } //e.g isNumeric(4.5) returns true
In this article, we have learnt how to validate decimal number in JavaScript. It can be used for data validation in your website or web applications.
Also read:
How to Fix Uncaught ReferenceError in jQuery
How to Detect Browser in JavaScript
How to Get Array Intersection in JavaScript
How to Sort Object Array by Date Keys
How to Pad Number With Zeroes in JavaScript
Related posts:
How to Count Substring Occurrence in String in JS
How to Detect Invalid Date in JavaScript
How to Detect Scroll Direction in JavaScript
How to Test for Empty JavaScript Object
How to Convert Comma Separated String into JS Array
How to Detect Touchscreen Device in JavaScript
How to Clear HTML5 Canvas for Redrawing in JavaScript
How to Get Value of Text Input Field With JavaScript
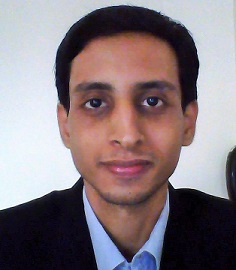
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.