Web developers and marketers employ numerous methods to increase and retain visitors on their websites. It is important to retain website visitors as long as you can, to increase conversion rate and grow your business. So, often web developers need to be able to detect when a user leaves their website or application, so they can try some ways to retain them and prevent them from leaving. In this article, we will learn how to detect when user leaves page using JavaScript. JavaScript is very useful to track user interaction such as checking if browser tab is active or detect user device.
Why Detect When User Leaves Page
There are several reasons why it is essential for web developers and administrators to figure out why users leave a page. First of all, it helps you understand whether the page design is intuitive and user friendly. If people are leaving the page immediately without clicking anything on it, then it means you need to re-design it better. It also helps you understand if users are responding to CTA (call to action) elements such as buttons and forms on your page. This is especially important if people are leaving your site’s checkout pages without completing the purchase. Otherwise, you may need to add/modify/remove them. You may also need to modify the text on your page to make it more relevant and interesting to your visitors. As you can see, there are several compelling reasons to keep track of how, why and when users leave your website.
How to Detect When User Leaves Page
You can implement this using plain JavaScript or using third-party JS libraries like jQuery. We will look at both these approaches. They both the use same method of listening to onbeforeunload event. When a user leaves a web page, this is the event that is automatically triggered by the web browser. So we need to create an event handler for it.
Most modern web browsers support onbeforeunload event that is automatically fired when user leaves a web page. It even allows you to ask user if they really want to leave. We will use this for our purpose.
1. Using Plain JavaScript
You can easily detect when user leaves your web page by simply adding the following JavaScript to your page.
<script language="JavaScript"> window.onbeforeunload = confirm_exit; function confirm_exit() { return "Are you sure you want to exit this page? All your unsaved changes will be lost."; } </script>
In the above code, we define an event listener function confirm_exit() that will be fired when event onbeforeunload is fired, when the user tries to leave the page. We have set up a warning message to be displayed to the user when confirm_exit() is called. You can customize this function as per your requirement. For example, you can use it to note the last scroll location of user.
Alternatively, you can also call this function when mouseleave event is fired. It is accurate in most cases, but not always.
<script language="JavaScript"> window.mouseleave = confirm_exit; function confirm_exit() { return "Are you sure you want to exit this page? All your unsaved changes will be lost."; } </script>
2. Using jQuery
JavaScript code can be a little complicated. If you are already using third-party JS libraries on your site, then you can also use them for this purpose. Most JavaScript libraries support event handling for onbeforeunload and mouseleave. For example, you can also do the same thing using third-party libraries like jQuery.
<script language="JavaScript"> $(document).bind("onbeforeunload", function(e) { confirm_exit(); }); function confirm_exit() { return "Are you sure you want to exit this page? All your unsaved changes will be lost."; } </script>
Here too you can bind the event listener to mouseleave event.
$(document).bind("mouseleave", function(e) { confirm_exit(); });
Conclusion
In this article, we have learnt a couple of simple ways to detect when user leaves a web page – using onbeforeunload and mouseleave events. Between the two, it is advisable to listen to onbeforeunload event. You can use it to detect and prevent users from leaving your website or web application. You can also use it to pause videos playing on your website, when user leaves the web page, and make your website for intuitive.
It is important to detect when and why users leave your web pages so that you can improve web design and improve user retention. If users leave your website without spending much time on it, then you will lose revenue. By tracking when user leaves web page, you can make a last ditch attempt to stop them from leaving or at least note the last location they were looking at, before they decided to leave.
Also read:
How to Generate PDF from HTML using JavaScript
How to Auto Resize IFrame Based on Content
How to Count Substring Occurrence in JS String
How to Convert Comma Separated String into JS Array
How to Suppress Warning Messages in MySQL
Related posts:
How to Check if JS Object Has Property
How to Check if Element is Hidden in JavaScript
How to Capitalize First Letter in JavaScript
How to Detect Invalid Date in JavaScript
How to Get Random Element from Array in JavaScript
How to Get URL Parameters Using jQuery or JavaScript
How to Read Local Text File in Browser Using JavaScript
How to Pad Number with Leading Zeroes in JavaScript
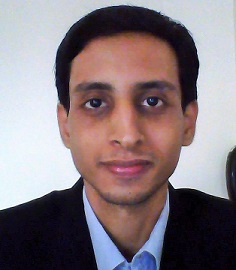
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.