Web developers often use strings to store data in their websites and applications. Often you may need to count substring occurrence in string in JS. There are several ways to do this. In this article, we will learn how to count substring occurrence in string in JS.
How to Count Substring Occurrence in String in JS
We will use match() function for our purpose. It returns an array of character or substring occurrence in string, based on a user-specific regular expression.
Let us say you have the following JS string.
var temp = "Hello World";
We will use the following match() function call to get an array of all occurrences of substring ‘el’ in above string.
temp.match(/el/g);
In the above function, we wrap the regular expression ‘el’ in /…/g to indicate it is a global regular expression, to search the whole string instead of just the first occurrence.
The above match() function will return an array of 1 element. If there are no matches, it will return null. So we will use the following command to get a count of substring occurrence in string.
var count = (temp.match(/el/g) || []).length; console.log(count); //prints 1 var count = (temp.match(/is/g) || []).length; console.log(count); //prints 0
Alternatively, you can also use split() function to split the given string by substring to obtain an array of substrings.
var temp = "This is a string."; console.log(temp.split("is").length - 1); //prints 1
The above command prints 1 because the split() function will split the string into an array of 2 elements, since ‘is’ occurs only once.
In this article, we have learnt a couple of simple ways to easily count substring occurrence in JS string. You can customize it as per your requirement.
Also read:
How to Convert Comma Separated String to JS Array
How to Suppress Warning Messages in MySQL
How to Show JavaScript Date in AM/PM Format
How to Get HTML Element’s Actual Width and Height
How to Call Parent Window Function from IFrame
Related posts:
How to Check if Element is Hidden in JavaScript
How to Create Multiline Strings in JavaScript
How to Use Variable As Key in JavaScript Object
How to Get data-id attribute in jQuery / JavaScript
How to Get Last Item in JS Array
How to Pass Parameter to SetTimeout Callback
How to Detect Touchscreen Device in JavaScript
How to Validate Decimal Number in JavaScript
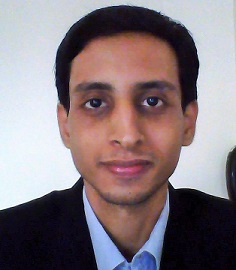
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.