JavaScript allows you to easily store data in arrays. JS arrays are one of the most widely used data structures due to their versatility and supported functions. Often, while working with arrays, you may need to get last item in JS array. In this article, we will learn how to do this.
How to Get Last Item in JS Array
While JS arrays provide many properties and functions, it is surprising that even after so many years of JS development there is no out-of-the-box function to get last element of array. Nevertheless, we will use commonly used approach to solve this problem. Let us say you have the following array.
var arr = [1, 2, 3, 4, 5];
Let us say you want to obtain the last item in JS array, that is, 5. Each JS array allows you to access its elements using indexes. It also provides length property that returns the number of elements in array. We will use both these information to calculate the index of last item of array as shown below. We use arr.length to get the number of elements in array. We subtract 1 from it, since array indexes start from 0 for 1st, element, 1 for 2nd element and so on.
arr.length - 1
Once we have the index of last item, we can easily obtain the item as shown below.
arr[arr.length - 1] //returns 5
Modern browsers also support at() function for each array, to return array element at a specific position. We can use -1 index value to obtain the last item of array.
arr.at(-1) //returns 5
In this article, we have learnt how to get last item of array using JavaScript.
Also read:
How to Check if Variable is Object in JS
How to Check if Browser Tab is Active
How to Find DOM Element using Attribute Value
How to Generate Hash from String in JavaScript
How to List Properties of JS Object
Related posts:
How to Pad Number with Leading Zeroes in JavaScript
How to Add Event to Dynamic Elements in JavaScript
How to Allow only Alphabet Input in HTML Text Input
How to Validate Decimal Number in JavaScript
How to Use JavaScript Variables in jQuery Selectors
How to Prevent Web Page from Being Loaded in Iframe
How to Add Delay in JavaScript Loop
How to Match Exact String in JavaScript
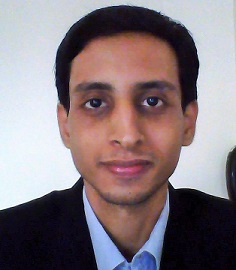
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.