JavaScript allows you to attach handlers to page elements to process various events. But most of these events are generally added to elements that are already present on your page. Sometimes you may need to add event handlers to dynamic elements in JavaScript. In such cases, the usual code will not bind the handler to your dynamic element, and will never be triggered. In this article, we will learn how to add event to dynamic elements in JavaScript.
How to Add Event to Dynamic Elements in JavaScript
Before we proceed, it is important to understand what is meant by dynamically generated page elements. Most web pages are dynamically generated using scripting languages such as PHP, Python, Ruby, etc. Please note, the page elements that are generated when these dynamic pages are rendered by your web server, are NOT dynamically generated elements. Dynamically generated elements are those elements that are added to an existing/loaded web page using JavaScript or JavaScript libraries such as jQuery, etc.
Let us say you want to add event handler to a dynamic element with id=’save_data’
<button id="save_data" />
Using JavaScript
Using JavaScript, here is the code to add event handler that is triggered when user clicks on this dynamically generated button.
document.addEventListener('click',function(e){ if(e.target && e.target.id== 'save_data'){ //do something } });
In the above code, we use document.addEventListener instead of element.addEventListener since our element is dynamically generated.
Within addEventListener we specify that the handler should be attached to ‘click’ event. Please note, this event will be called no matter where you click on the document. So, within the even handler for this click event, we check the id of target, that is, the clicked element. Only if it matches our element’s id (save_data), we execute further code.
Using Jquery
You can also use jquery library for this purpose, which is a much easier way to solve this problem. Here is the code to handle click event on dynamically generated element with id=save_data.
$(document).on('click','#save_data',function(){//do something})
Here also we use on() function to bind the click event to the document, instead of element. It allows you to specify which element or elements you need to handle the event for, as the second argument. So we specify it as #save_data. Since we are specifying the id of element we add hash(#) at the beginning of identifier. If you want to attach the event to a bunch of dynamically generated elements, such as multiple buttons, all identified by the same class (e.g. ‘submit_data’) then you need to use dot(.) before the classname, instead of using hash(#).
$(document).on('click','.submit_data',function(){//do something})
Please note, if you are using Jquery, you will need to first include the Jquery library on your web page so that its functions work. Here is a simple example to do this.
<head> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script> </head>
In this article, we have learnt a couple of ways to add event to dynamic elements in web pages, using plain JavaScript. It is very useful in adding event listeners to dynamically generated elements.
Also read:
How to Clone Objects in JavaScript
How to Replace All Occurrences of String in JavaScript
Python String Slicing
How to Select Rows from DataFrame Using Column Names
How to Rename Column in Python Pandas
Related posts:
How to Return Response from Asynchronous Call in JavaScript
How to Compare Arrays in JavaScript
How to Get URL Parameters Using jQuery or JavaScript
How to Format Number as Currency String
How to Change Element's Class in JavaScript
How to Attach Event to Dynamic Elements in JavaScript
How to Check if Variable is Array in JavaScript
How to Get Value of Text Input Field With JavaScript
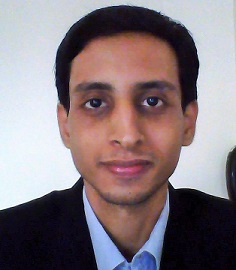
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.