jQuery allows you to easily bind events to all kinds of DOM elements on your web page and perform various actions when those events are triggered. But in most cases, these event handlers such as click(), blur(), etc. work with elements that are static and not dynamically generated. You will realize that when you try to attach an event to an element, jQuery will not allow you to do this. In this article, we will learn how to bind event to dynamic element in jQuery.
How to Bind Event to Dynamic Element in jQuery
Let us say you have a statically created element on your page as shown below. In other words, this element’s HTML is already present on your web page’s HTML or template so it is not dynamically rendered.
<button id='myButton'>Click</button>
Typically, if we want to bind click event to this element, we will do something like the following.
$('#myButton').click(function(e){ ... })
The above approach works since our DOM element is static.
Here is an example of dynamically created using the following kind of code. We create the same button but dynamically this time.
$( document ).ready(function() { $('body').append('<button id='myButton'>Click</button>'); });
Now if you try binding the click event to above generated button DOM element it will not work, because click() function binds only to static elements and not dynamic ones. In this case, our button element is generated only after the page is loaded.
In such cases, you need to use the on() function to bind events to dynamic elements. Here is its syntax. It is supported in jQuery 1.7 or later.
$(staticAncestors).on(eventName, dynamicChild, function() {});
The above command works as follows. The event is attached to a static ancestor staticAncestors (such as document, body, etc.) of the dynamic element. The event handler is called every time the event is triggered on the static element or its descendants. The handler will check if the element that triggered the event matches the selector dynamicChild. If so, its event handler function() is called.
Here is the command to bind click event to our dynamic element.
$(document).on('click', '#myButton',function(){...});
In the above command, we bind on() event to document. We specify the event name as click, so that it is triggered only when someone clicks any part of document. The second argument specifies the selector of the element to which we want to bind the click event. Whenever someone clicks document, it will check if the click was performed on element with ID=myButton. If so, it will execute the corresponding event handler function.
It is advisable to pick the nearest static ancestor of dynamic element (such as div), instead of using generic static elements like document or body, since this will trigger too many events and might not be efficient. For example, if you bind on() function to document, then every time there is a click on any part of HTML document, the handler will check if it was performed by myButton, which is not efficient.
If you are using jQuery < 1.7, then you need to use live() function which has the following syntax.
$(selector).live( eventName, function(){} );
Here is an example of using live() function to attach click event to dynamic element myButton.
$('#myButton').live( 'click', function(){...} );
In this article, we have learnt how to bind events to dynamic elements. You can use them to bind all kinds of events such as blur, mouseUp, mouseDown, etc. not just click events.
Also read:
How to Find Sum of Array of Numbers in JavaScript
Remove Unicode Characters from String
Remove Diatrics/Accents from String in Python
Remove Diatrics/Accents from String in JavaScript
How to Convert Array to JS Object in JavaScript
Related posts:
How to Allow only Alphabet Input in HTML Text Input
How to Use Dynamic Variable Names in JavaScript
How to Validate Decimal Number in JavaScript
How to Remove Duplicates from Array of Objects JavaScript
How to Check if Object is Empty in JavaScript
How to Empty Array in JavaScript
How to Prevent Page Refresh on Form Submit
How to Get Position of Element in HTML
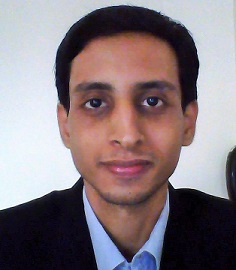
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.