JavaScript Arrays and Objects are two popular data structures used extensively by web developers to store their data. Often we need to interchange them from one form to another. In this article, we will learn how to convert array to object in javaScript.
How to Convert Array to Object in JavaScript
ECMAScript 6 onwards, you can use Object.assign() function to convert array to object. It is basically used to copy data from one or more enumerable data to a target object. Here is an example to convert array [‘a’, ‘b’, ‘c’] to {0:”a”, 1:”b”, 2:”c”}.
Object.assign({}, ['a','b','c']);
Please note, in this case, assign() function will automatically assign key values as 0, 1, 2 for 1st, 2nd 3rd element of your array.
Alternatively, you can also use spread operator (…) to convert array into JS object. This is supported in ECMAScript 8, that is, modern web browsers.
{ ...['a', 'b', 'c'] }
If you want to assign custom keys, you can use reduce() function. Here is an example to use keys a, b, c for values a, b, c respectively.
['a', 'b', 'c'].reduce((a, v) => ({ ...a, [v]: v}), {}) // { a: "a", b: "b", c: "c" }
In this article, we have learnt how to convert array to object in JavaScript. It is very useful to quickly interchange data types from one format to another.
Also read:
How to Convert RGB to Hex and Hex to RGB
How to Store Emoji in MySQL Database
How to Select Nth Row in Database Table
How to Search String in Multiple Tables in Database
How to Split Field into Two in MySQL
Related posts:
How to Change Href Attribute of Link Using jQuery
How to Replace Line Breaks With <br> in JavaScript
How to Remove All Child Elements of DOM Node in JS
How to Disable Clicks in IFrame Using JavaScript
How to Get Nested Object Keys in JavaScript
How to Compare Arrays in JavaScript
How to Check if JavaScript Object Property is Undefined
How to Store Data in Local Storage in JavaScript
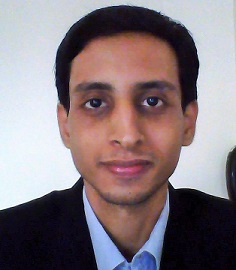
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.