JavaScript object is a powerful data structure that allows you to easily store different data types in a compact manner. It is advisable to check if JS object is empty before you use it. Otherwise, your JS code may throw an error and stop code execution. In this article, we will learn how to test for empty JavaScript object in different JavaScript languages and libraries.
How to Test for Empty JavaScript Object
Let us say you have the following JS object.
obj
Here is the plain JavaScript code to check if it is empty or not.
obj // null and undefined check && Object.keys(obj).length === 0 && Object.getPrototypeOf(obj) === Object.prototype
The above code consists of 3 checks. The first one checks if the object is null/undefined. The second check tests if it has no keys (empty). The third one checks if it is actually an object data type or some other data.
If the result of above expression is true then the JS object is empty, else it is not.
If you use jQuery, then you can easily check if a JS object is empty or not using isEmptyObject() function. It returns true if the passed object is empty, else it returns false.
jQuery.isEmptyObject(obj); // true
If you use popular JS libraries like Lodash or Underscore, you can isEmpty() function to check if an object it empty or not.
_.isEmpty(obj); // true
If you use ExtJS library, you can use its isEmpty() function as shown below.
Ext.Object.isEmpty(obj); // true
With AngularJS you need to compare the JS object with an empty object to check if it is empty or not.
angular.equals(obj, {}); // true
In this article, we have learnt how to easily check if a JS object is empty or not, using plain JavaScript as well as various JS libraries.
Also read:
How to Convert RGB to Hex and Hex to RGB in JavaScript
How to Store Emoji in MySQL Database
How to Select Nth Row in Database Table
How to Search String in Multiple Tables
How to Split Field into Two Columns in MySQL
Related posts:
How to Get Class List for DOM Element
How to Access Iframe Content With JavaScript
How to Remove Element By Id in JS
How to Compare Two Dates Using JavaScript
How to Get Previous URL in JavaScript
How to Add Days to Date in JavaScript
How to Get Property Values of JS Objects
How to Check if Browser Tab is Active
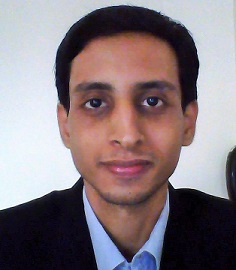
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.