Each DOM element can have multiple classes assigned to it. Often web developers need to get the list of all class names that are assigned to one or more elements on their page, so that they can perform some tasks based on specific class names. There are several ways to do this using JavaScript as well as using third party libraries like jQuery. In this article, we will learn how to get a class list of DOM element.
How to Get Class List for DOM Element
Let us say you have the following div with multiple classes.
<div id='myDiv' class='class1 class2 class3'>...</div>
Here is a simple JavaScript code snippet to get a list of all class names of a DOM element as an array.
var classList = document.getElementById('myDiv').className.split(/\s+/); for (var i = 0; i < classList.length; i++) { if (classList[i] === 'someClass') { //do something } }
In the above code, we select our div using its ID value as selector and getElementById() function. Each DOM element supports className function that returns the complete string used in class attribute. We call split() function on this string to split it by whitespace characters, since classes are separated by whitespace characters. This will return an array of class names that you can iterate using a for loop or some other way and use them as per your requirement.
If you are using jQuery, you can modify the above code as shown below. Basically the logic remains the same, but just the commands have been changed to suite jQuery.
var classList = $('#myDiv').attr('class').split(/\s+/); $.each(classList, function(index, item) { if (item === 'someClass') { //do something } });
If you are using modern browsers like Chrome or Firefox, you can also use classList attribute that automatically returns an array of classes.
var list = document.getElementById("myDiv").classList;
You can also add more classes to this list if you want, as shown below.
list.add("class4");
In this article, we have learnt how to get class list for DOM element. It is useful in fetching all class names attached to a DOM element so you can work with them as per your requirement.
Also read:
How to Automatically Scroll to Bottom of Page in JS
How to Change Image Source in jQuery
How to Check for Hash in URL Using JavaScript
How to Check if File Exists in NodeJS
How to Load Local JSON File
Related posts:
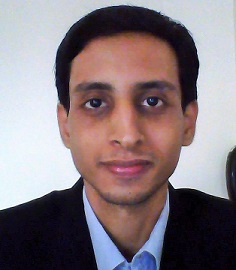
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.