By default, when a user refreshes or reloads a web page, all the variables on that page are reset and reassigned data. But sometimes you may need to persist variables between page load. For example, you may want to keep track of the current active tab on your page, and retain it even when user refreshes their page. In this article, we will learn how to persist variable between refresh or keep values after page reload. You can use it to store data on client side of your website or web application.
How to Persist Variables Between Page Load
There are two ways to persist variables between page load.
1. Using Query String
One of the simplest ways to preserve the values of variables on page load is to pass them as query string in the requested URL. For example, let us say you want to load http://example.com/home.html and your variable ‘a’ has value 1 on this page. When a user clicks a button to refresh page or otherwise, you can request URL as http://example.com/home.html?a=1 instead of requesting just http://example.com/home.html. In this case we have included the variable with its value as query string in requested URL.
On the page displayed for above URL, add the following code.
const params = new Proxy(new URLSearchParams(window.location.search), { get: (searchParams, prop) => searchParams.get(prop), }); // Get the value of "key a" in eg "https://example.com/home.html?a=1" let value = params.a; // "1"
The above code parses all query string variables into a JS object. Now you can assign the above value to variable on your page to retain it.
var a=value;
2. Using Web Storage
The above technique works for GET requests but if you want to persist variables across POST requests, or if you have a lot of data to be stored, then you need to use web storage.
With HTML5, browsers offer localStorage and sessionStorage objects that allow you to store data locally which can be retrieved later when you refresh the page or go to another page. localStorage stores your data until you clear it manually or programmatically while sessionStorage stored data only as long as your current session is in progress.
Both localStorage and sessionStorage store information as key-value pairs.
Here is the syntax to store data in them using setItem() function.
sessionStorage.setItem(variable, value); localStorage.setItem(variable, value);
Here are a couple of examples of the same.
sessionStorage.setItem('clicked', true); localStorage.setItem('checked', true);
Here is the command to retrieve variables from them using getItem() function and store them in variables.
var clicked = sessionStorage.getItem('clicked'); var checked = sessionStorage.getItem('checked');
Here is the command to remove these key-value pairs programmatically.
sessionStorage.removeItem('clicked'); localStorage.removeItem('checked');
3. Using Cookies
Although cookies are mainly meant for servers, you can also use it to store client side data. Here is a simple way to store information using cookies in jQuery.
$.cookie(variable, value, time_limit);
Here is an example.
$.cookie('clicked', 'true', {expires: 1}); // expires in 1 day
Here is how to store data in cookie using plain JavaScript.
document.cookie = "clicked=true; expires=Thu, 18 Dec 2022 12:00:00 UTC";
In this article, we have learnt several ways to persist variables between page load. You can use any of these methods depending on your requirement.
Also read:
How to Scroll to Element With JavaScript
How to Scroll to Element With jQuery
How to Add Delay in JavaScript Loop
How to Use Dynamic Variable Names in JavaScript
How to Get Difference Between Two Arrays in JavaScript
Related posts:
How to Check if Element is Hidden in JavaScript
How to Add Delay in JavaScript Loop
How to Get Random Element from Array in JavaScript
How to Compare Two Dates Using JavaScript
How to Add Days to Date in JavaScript
How to Remove Duplicates from Array of Objects JavaScript
How to Check if Variable is Array in JavaScript
Return False vs PreventDefault in JavaScript
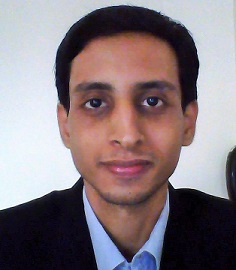
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.