Typically, JavaScript variable names are literal strings of alphanumeric characters. Sometimes you may need to use dynamic variable names in JavaScript. In this article, we will learn how to use dynamic variable names in JavaScript.
How to Use Dynamic Variable Names in JavaScript
We will look at a couple of ways to use dynamic variable names in JavaScript.
One of the most common ways of using dynamic variable names is via eval function, which evaluates any expression and returns its result. Let us say you have the following variable.
a=1;
Next, let us say you create another variable b.
b='a';
Now let us say you want to print the value of variable a using variable b’s value. If you directly use variable it will only print string ‘a’ and not the variable a’s value.
console.log(b);//displays 'a'
This is because, in the above case, b is treated as a variable and its value is literally displayed. Here is how to overcome this problem using eval() function.
console.log(eval(b));//displays 1
However, it is advisable to avoid using eval since it blindly evaluates any expressions passed to it. So if somebody manages to modify your variable, they can get eval to evaluate malicious code on your page.
Another way to do this is using the scope of window object. Every variable that you define on your web page is stored in window object. Let us say you have the following JS variable.
var a=1;
As mentioned earlier, the following line will not assign variable a’s value to variable b only the string ‘a’ to variable b.
b='a';
In this case, you can assign a’s value to variable b the following way.
var b = window.a; OR var b = window['a'];
Now when you display b’s value you will find that it contains variable a’s value.
console.log(b);//prints 1
Similarly, you can access the value of variables defined inside a function using this keyword. For example,
function def(){ var b = 1; console.log(this.b); }
In this article, we have learnt how to use dynamic variable names.
Also read:
How to Get Difference Between Two JS Arrays
How to Compare Dictionary in Python
How to Compare Objects in JavaScript
How to Group By Array of Objects by Key
How to Find Element Using Xpath in JavaScript
Related posts:
How to Attach Event to Dynamic Elements in JavaScript
How to Convert Array to Object in JavaScript
How to Check if JS Object Has Property
How to Get Highlighted/Selected Text in JavaScript
How to Change Element's Class in JavaScript
How to Render HTML in TextArea
Remove Unicode Characters from String
How to Empty Array in JavaScript
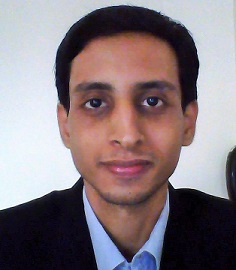
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.