Python dictionary is a data structure that allows you to store same or different types of data in a compact manner as key-value pairs. They are often used by developers to retrieve and store data from front end of websites and applications. Often you may need to compare dictionary in Python. There are several simple ways to do this in python. In this article, we will learn a couple of ways to compare dictionary in Python.
How to Compare Dictionary in Python
Let us say you have the following 3 python dictionaries.
dict1 = {'Name': 'Jim', 'Age': 5} dict2 = {'Name': 'Jim', 'Age': 5} dict3 = {'Name': 'John', 'Age': 50}
1. Using Equality Operator
You can easily check if two dictionaries are equal or not using the equality operator == as shown below.
>>> dict1 == dict2 True >>> dict2 == dict3 False
2. Using DeepDiff Module
DeepDiff is a useful third party module that allows you to easily compare dictionaries, iterables, strings, and other objects. Here is the command to install it.
$ pip install deepdiff
Once it is installed, you can easily compare two python dictionaries using the following command.
from deepdiff import DeepDiff diff1 = DeepDiff(dict1, dict2) print(diff1) # returns True diff2 = DeepDiff(dict1, dict3) print(diff2) # returns False
In this article, we have learnt how to compare dictionaries in Python. You can use it in another python function or module as per your requirement.
Also read:
How to Compare Objects in JavaScript
How to Group By Array Of Objects by Key
How to Find Element by XPath in JavaScript
How to Remove Submodule in Git
How to Merge Two Git Repositories
Related posts:
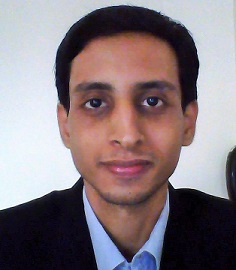
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.