Sometimes you may need to read or accept user input in python. This is a common requirement in applications which require user interaction. There are many ways to do this, most of which require a GUI dialog box. But if your application is a command line based then you can easily accept user input via keyboard using the following commands. In this article, we will look at how to read user input in python via keyboard prompts.
How to Read User Input in Python
Here are the different ways to accept user input in python.
1. Using input()
input() function allows you to accept both string as well as numbers from user input. It accepts the user input text and then parses it to determine if it is a string or number. If the user does not provide a right input, it raises an exception.
# example using input() val = input("Enter input: ") print(val)
When you run the above script, it will look like
Enter input:abc abc
Please note, during execution, when python comes across input() function it will pause the program execution till user submits input. You don’t need to enter a string in input function, it is optional. If you don’t enter any string, the input function will simply pause execution and wait for user input at that point. Since this might confuse the user, it is advisable to enter a string asking user to enter input.
Also, in python 2.x, whatever user enters, number or alphabets, python will convert it into a appropriate data type. For example, if you enter an integer, it will store it in an int, if you enter a string, it will store it as string variable, if you enter float, then python will store it as floating point variable. Here is an example.
Here is an example.
>>> val=input("enter input:") enter input:123 >>> type(val) <type 'int'> >>> val=input("enter input:") enter input:'abc' >>> type(val) <type 'str'> >>> val=input("enter input") enter input123.34 >>> type(val) <type 'float'>
Also remember to put single/double quotes around alphabets or alphanumeric strings. Otherwise python will throw an error.
>>> val=input("enter input:") enter input:abc Traceback (most recent call last): File "<pyshell#2>", line 1, in <module> val=input("enter input:") File "<string>", line 1, in <module> NameError: name 'abc' is not defined
Please note, since python 3+, whatever you enter as user input for input() function, python will convert it into string and store it. You need to explicitly convert it into the data type (int, float, etc) you want, after receiving user input.
2. Using raw_input
This function is available in python 2.x only. It accepts user input and converts it into string and returns it to the variable, in case we want to store it. Otherwise, it will display the string value in command prompt.
>>> a=raw_input('enter input:') >>> enter input:123 >>> a '123' >>> type(a) >>> <type 'str'>
In this article, we have looked at two different ways to accept user input. As mentioned earlier, reading user input is generally part of large applications and scripts in python. input() function allows you to easily accept user input no matter whether it is a number, characters, or strings.
Also read:
How to Empty List in Python
How to Open Image in Terminal
Bash Sort By Column
How to Configure PAM in Linux
How to Read Large CSV File in Python
Related posts:
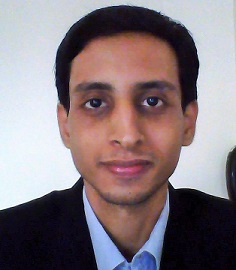
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.