Python allows you to process a wide range of files such as text files, csv files and even images. Sometimes you may need to read binary file in python. In this article, we will look at how to read binary file in python. We will also look at different use cases of reading binary file that are commonly used by developers.
How to Read Binary File in Python
Here is how to read binary file in python. Let us say you have a binary file at /home/ubuntu/data.bin. First we will write some data into our binary file. For that, you can open the file using open function with wb flag.
file = open("/home/ubuntu/data.bin","wb") sentence = bytearray("Hello world".encode("ascii")) file.write(sentence) file.close()
We use encode function to first encode our string into ascii character encoding. Then we use bytearray function to convert into a byte array. We use file.write function to write into the file and file.close function to close the file.
Please note, you can read/write any file, not just, .bin files, but also .txt, .csv or any other file as a binary file. When you specify rb and wb flags with open command, python will automatically read and write the file in binary mode.
Read bytes from Binary File
Now you can easily read the file using read function.
file = open("/home/ubuntu/data.bin","rb") print(file.read(4)) file.close()
In the above code, we open the file using open function and rb flag. Then we use read function to read 4 bytes from it, that is, 4 characters. We use print function to print it and finally close the file. Here is the output of above command.
Hell
Read Binary File to Array
Sometimes you may want to read bytes from binary to an array. In such cases you can easily do this using list() function as shown below.
file = open("/home/ubuntu/data.bin","rb") arr=list(file.read(4)) print(arr) file.close()
In the above code, we open the file in binary mode to read. Then use read function to read the first 4 bytes. We pass it to list() function to convert the string into an array. Here is the output of the above code
['H','e','l','l']
Python read binary file line by line
Many times you may need to read binary file line by line. In this case, we first write multiple lines into our file using writelines function as shown.
lines=["Welcome to fedingo","Good Morning"] file=open("/home/ubuntu/data.bin","wb") file.writelines(lines) file.close()
The above code will write 2 lines into our file. Now we will open our file again for reading in binary mode, and use readline function to read a line from it.
file=open("/home/ubuntu/data.bin","rb") line=file.readline() print(line) file.close()
Here is the output of above command.
Welcome to Fedingo
In this article, we have looked at various ways to read & write binary files. It is important to note that you can open any file as binary file, using rb and wb flags for reading & writing respectively. Once you have opened the file, use read() function to read bytes from and write() function to write bytes to your binary file respectively. Also, you may use readline() function to read lines from and writelines() to write lines to your binary file respectively.
Also read:
How to Read User Input in Python
How to Empty List in Python
How to Open Image in Terminal
Bash Sort by Column
How to Configure PAM in Linux
Related posts:
How to Split Python List into N Sublists
How to Convert Columns into Rows in Pandas
How to Concatenate Multiple Lists in Python
How to Check if File Exists in Python
How to Count Occurrence of List Item in Python
How to Create Nested Directory in Python
How to Password Protect PDF in Python
How to Terminate Python Subprocess
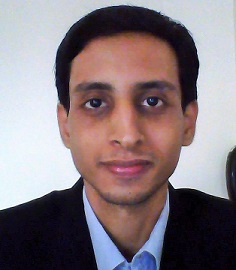
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.