Python allows you to store different types of data in a single place using lists. It also provides many features for list manipulation. However, sometimes you may need to delete list items, clear list in python or remove list elements. In this article, we will look at how to empty list in python. Python offers many functions and constructs to clear a list. We will look at each of them one by one.
How to Empty List in Python
Here are the different ways to empty list in python.
1. Using clear()
You can directly call clear method on any python list as shown below.
#create list a=[1,2,3] #clear list a.clear() #display list a []
2. Reinitialize List
In this case, we simply reinitialize the list with no values, thereby deleting its contents. Here is an example.
#define list a=[1,2,3] #reinitialize list a=[] #display list a []
3. Using assignment
This is not a well-known method but it also gives the same result as the above methods to clear a list.
#create list a=[1,2,3] #clear list a*=0 #display list a []
4. Using del command
del command can also be used to clear a list, or delete its elements as shown below.
#create list a=[1,2,3] #delete list del a[:] #display list a []
Please note, delete command can be used to delete one or more elements, not just emptying it. Here is an example to delete only the second element in the list.
#create list a=[1,2,3] #delete second element del a[1] #display list a [1,3]
In this article, we have looked at how to delete, empty or clear a python list. You may use any of these methods as per your requirement.
Deleting list is a frequent requirement especially in python-based applications and web sites where you want to clean up a list and add new elements to it. Generally, the above codes are part of larger functions and modules where you need to reset lists to new values. If you don’t want to delete the entire list but only a part of it then you may also use functions like pop or slice operators to reassign the list with required elements.
Also read:
How to Open Image in Terminal
Bash Sort By Column
How to Configure PAM in Linux
How to Read Large CSV File in Python
How to Get Filename from Path in Python
Related posts:
How to Print in Same Line in Python
How to Check Version of Python Modules
How to Count Repeated Characters in String in Python
How to Comment in Python
How to Convert DocX to Pdf in Python
How to Convert PDF to Text in Python
How to Rename File Using Python
How to Redirect Stdout & Stderr to File in Python
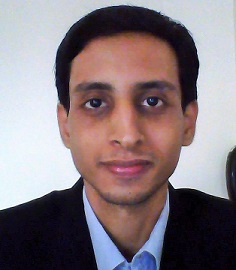
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.