A file path is a string that uniquely identifies the location of file on file system. Sometimes you may need to retrieve or extract filename from file path in python. There are various ways to do this python. In this article, we will look at how to get filename from path in python.
How to Get Filename from Path in Python
We will look at different ways to get filename from path in python. We will use os.path and pathlib libraries for this purpose.
1. Using os.path.basename
You can easily get filename using os.path.basename function. It will return the filename with extension. This method works for almost every python version.
In Windows
>>> import os >>> fpath='c:\Project\data.txt' >>> os.path.basename(fpath) 'data.txt'
In Linux
>>> fpath="/home/ubuntu/data.txt" >>> import os >>> os.path.basename(fpath) 'data.txt'
Please note, filepaths in Windows contain back slash while those in Linux contain forward slash. However, in both cases, python will correctly parse the file path and return the filename.
If you don’t want the file extension but only the filename, use os.path.splitext function to split the filename and extension separately into an array. Use index 0 to get the filename without extension. Here are the above examples with os.path.splitext.
In Windows
>>> import os >>> fpath='c:\Project\data.txt' >>> base=os.path.basename(fpath) >>> print base data.txt >>> fname=os.path.splitext(base)[0] >>> data
In Linux
>>> fpath="/home/ubuntu/data.txt" >>> import os >>> base=os.path.basename(fpath) >>> print base data.txt >>> fname=os.path.splitext(base)[0] >>> data
If you want to extract only filename from path, without extension, you need to first use os.path.basename to get the filename with extension, and then use os.path.splitext split the filename from extension. If you directly call os.path.splitext on filepath, then it will split extension from filepath, without extracting the filename. Here is an example of it.
>>> fpath="/home/ubuntu/data.txt" >>> import os >>> fname=os.path.splitext(fpath)[0] >>> /home/ubuntu/data
Please note, the splitext method will only split the last extension. For example, if your filename is data.tar.gz then splitext will return data.tar, instead of returning data. In case, your filename has multiple extensions and you want to get only the filename, then you need to pass output of one splitext function to another.
>>> fpath="/home/ubuntu/data.tar.gz" >>> import os >>> base=os.path.basename(fpath) >>> base data.tar.gz >>> fname=os.path.splitext(base)[0] data.tar >>> fname =os.path.splitext(fname)[0] data
2. Using pathlib
Starting python 3, you can also use pathlib library to easily extract filename from path. In fact, it is available as a part of standard library starting python 3.4. Here is an example
In Windows
>>> from pathlib import Path >>> fpath='c:\Project\data.txt' >>> Path(fpath).stem data
In Linux
>>> from pathlib import Path >>> fpath="/home/ubuntu/data.txt" >>> Path(fpath).stem data
Please note, the .stem above will only remove the last extension. For example, if your file name is data.tar.gz, then .stem will return data.tar.
In such cases, you have to pass the output of one Path statement, to the other.
>>>Path(Path(/home/ubuntu/data.tar.gz).stem).stem data
In this article, we have looked at two different ways to get filename from path in python. They are both useful and adapt well to Windows-style paths as well as Linux-style paths.
Both os.path and pathlib provide useful functions to get filename from path. os.path works on all Python versions while pathlib is available in python 3+. In fact, it was created explicitly to work with filepaths and directory paths. However, if your filename contains multiple extensions, you will have to repeatedly split it to extract only the filename. Nevertheless, you may use either of them depending on your requirement.
Also read:
How to Increase SSH Connection Timeout
How to Run Sudo Command Without Password
How to Save Dictionary to File in Python
How to Kill Unresponsive Process in Linux
How to Lock User After Failed Login Attempt
Related posts:
How to Import from Another Folder in Python
How to Get Field Value in Django Queryset
How to Randomly Select Item from List in Python
How to Terminate Python Subprocess
How to Iterate Through List of Dictionaries in Python
How to Flatten List of Dictionaries in Python
How to Get File Size in Python
How to Schedule Task in Python
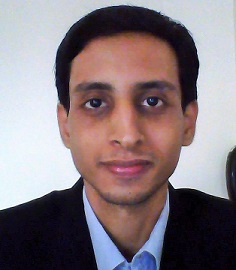
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.