Sometimes you may need to write python dictionary to file. This process of writing various python data structures such as lists, tuples, dictionaries and objects to file is also known as pickling. In this article, we will look at how to save dictionary to file in python. For our examples, we will consider most common use cases such as writing dictionary to csv, txt and json file types.
How to Save Dictionary to File in Python
Here are the different ways to write dictionary to file in python.
1. Save dictionary to csv file
We will use csv module to write dictionary to csv file. In this case, we will get one key-value combination on each line of csv file, separated by comma. Here is an example for it.
# dict_to_csv.py import csv dict_sample = {'name': 'fedingo', 'city': 'NYC', 'education': 'Engineering'} with open('data.csv', 'w') as f: for key in dict_sample.keys(): f.write("%s, %s\n" %(key, dict_sample[key]))
In the above code, we import csv module and define a dictionary dict_sample to be written to file data.csv. Next, we use with statement & open function to open the csv file for writing. We use a for loop to iterate through the key-value pair of dictionary. In each iteration, we use write function to write the key and value to data.csv file. While writing them we mention a format specifier such that key and value are separated by comma, and the value is followed by newline character.
When you run the above file
$ python dict_to_csv.py
you will get the following output
name, fedingo city, NYC education, Engineering
2. Save Dictionary to JSON
In this case, we will write dictionary to JSON file. For this purpose, we import JSON module which provides a very convenient function JSON.dumps to directly write dictionary to JSON format. In fact, you can use this function to convert any kind of python data into JSON format, whether it is list, tuples, dictionaries or just strings.
Here is an example to save python dictionary to JSON file.
# dict_to_json.py import json dict_sample = {'name': 'fedingo', 'city': 'NYC', 'education': 'Engineering'} with open('data.json', 'w') as f: json.dump(dict_sample, f)
In the above example, we first import JSON module. Then we define a dictionary for writing to JSON file. We use with statement and open command to open data.json file for writing. Finally, we use json.dumps command to write dictionary to JSON file.
Now if you view content of your json file, it will look as follows.
$ cat data.json {"name": "fedingo", "city": "NYC", "education": "Engineering"}
You will notice that in the above JSON output, all single quotes in dictionary have been converted into double quotes in JSON, confirming the data conversion.
3. Save Dictionary to Text File
In this case, we will be writing dictionary to text file. There are two ways to do this – either you can directly the entire dictionary as-is to text file, or you can parse the dictionary and write each key value on new line, separated by tabs, just as we did for csv file (section #1) above. Let us look at both these cases.
The following code will write python dictionary as-is to text file.
# dict_to_txt.py dict_sample = {'name': 'fedingo', 'city': 'NYC', 'education': 'Engineering'} with open('data.csv', 'w') as f: f.write(str(dict_sample))
In the above example, we do not need to import any module since python supports txt file operations out of the box. We simply use with and open commands to open the file for writing, and use write command to write the dictionary. We use str command to convert dictionary into a string before writing it, since you cannot write dictionary directly to files.
Now if you view content of your txt file, it will look as follows.
$ cat data.txt {"name": "fedingo", "city": "NYC", "education": "Engineering"}
The following code will parse you python dictionary and convert it into tab delimited text file that you can easily import into other applications.
# dict_to_txt.py dict_sample = {'name': 'fedingo', 'city': 'NYC', 'education': 'Engineering'} with open('data.txt', 'w') as f: for key in dict_sample.keys(): f.write("%s %s\n" %(key, dict_sample[key]))
In the above code, define a dictionary dict_sample to be written to file data.txt. Next, we use with statement & open function to open the txt file for writing. We use a for loop to iterate through the key-value pair of dictionary. In each iteration, we use write function to write the key and value to data.txt file. While writing them we mention a format specifier such that key and value are separated by space/tab, and the value is followed by newline character.
Here is the output data.txt file
name fedingo city NYC education Engineering
That’s it. In this article, we have learnt how to save dictionary to file. While doing so, we have looked at the 3 most common use cases of writing dictionary to csv, txt and json files. Writing dictionaries are generally parts of large functions and modules in applications. You may customize and use it in your application, as per your requirement.
Also read:
How to Kill Unresponsive Process in Linux
How to Lock User After Failed Login Attempts in Linux
How to Find Failed SSH Login Attempts in Linux
How to Schedule Shutdown in Linux
How to Secure SSH Server in Linux
Related posts:
How to Show Progress in Rsync
How To Search in VI Editor
How to Revoke SSH Access & Keys in Linux
How to Use SCP with PEM File (SSH Key)
How to Restore Default Repositories in Ubuntu
How to Fix dpkg Was Interrupted, You Must Manually Run Sudo
How to Set SSH Warning Message in Linux
How to Truncate File in Linux
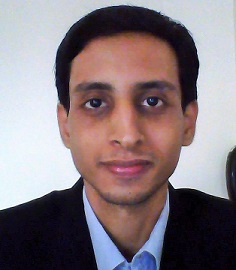
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.