Sometimes you may need to schedule task in python applications & services, to run them at specific times. Typically, developers and system administrators use cronjob in Linux/Unix and Scheduled Tasks in Windows to schedule tasks and processes. In this article, we will learn how to schedule task in Python. For this purpose, we will use schedule library that
How to Schedule Task in Python
Schedule module allows you to run python functions at specific times and intervals using simple syntax. You can use it to run a tasks once, at specific times of a day, or on specific days of week. It basically matches system time with the scheduled time and runs tasks when the scheduled time arrives.
Here is the command to install Schedule module.
$ pip install schedule
It mainly provides two classes – schedule.Scheduler and schedule.Job each of which provides several useful functions. Let us look at them in detail.
Here are the functions supported by schedule.Scheduler class.
schedule.every(interval=1)
: Schedules a new job.schedule.run_pending()
: Runs all pending jobs that are scheduled to run.schedule.run_all(delay_seconds=0)
: Run all jobs regardless whether they are scheduled to run now or not.schedule.idle_seconds()
: Sets idle time on the default scheduler instance.schedule.next_run()
: Runs next job scheduled to run.schedule.cancel_job(job)
: Deletes a scheduled job.
Here is the command to schedule a job
schedule.Job(interval, scheduler=None) class
interval: A quantity of a certain time unit
scheduler: Scheduler instance to handle this job
Here are the basic methods to schedule a job.
at(time_str)
: Schedule a job to run every day at a specific time.do(job_func, *args, **kwargs)
: Specifies the function job_func to be called every time the job runs.run()
: Run the job and immediately schedule it.to(latest)
: Schedule the job to run at randomized intervals.
Here is an example to use schedule library to schedule functions.
import schedule, time # Functions setup def hello_world: print("Hello World") # Task scheduling # After every 10mins hello_world() is called. schedule.every(10).minutes.do(hello_world) # After every hour hello_world() is called. schedule.every().hour.do(hello_world) # Every day at 12am or 00:00 time hello_world() is called. schedule.every().day.at("00:00").do(hello_world) # After every 5 to 10mins in between run hello_world() schedule.every(5).to(10).minutes.do(hello_world) # Every monday hello_world() is called schedule.every().monday.do(hello_world) # Every tuesday at 18:00 hello_world() is called schedule.every().tuesday.at("18:00").do(hello_world) # Loop so that the scheduling task # keeps on running all time. while True: # Checks whether a scheduled task # is pending to run or not schedule.run_pending() time.sleep(1)
In the above code, we first import schedule and time library. Schedule library is used to manage scheduled tasks and time library is used to call sleep function, that runs scheduler after every 1 second to check if there are any pending scheduled tasks. The scheduler library also provides a default instance used to schedule tasks.
We have defined a simple hello_world() function that we will call in different schedules. Then we have used the scheduler instance to run hello_world() function after every 10 minutes, every hour, everyday at midnight, every 5-10 minutes, every Monday and every Tuesday.
Finally, we run a never ending while loop that calls schedule.run_pending() function after every 1 second. This function checks the system time against pending scheduled tasks and run the tasks whose time has come.
In this article, we have learnt how to schedule tasks in python. You can use it to schedule tasks from within python script, applications, services, as per your requirement. Alternatively, you can also use threading.Timer() function, which is available by default in python, to schedule tasks but scheduler library is much more powerful and offers better control over scheduled tasks.
Also read:
How to Import Python Modules by String Name in Python
Call Python Function by String Name
How to Save Git Username & Password
How to Get Classname of Instance in Python
How to Lock File in Python
Related posts:
How to Extract Numbers from String in Python
How to Drop One or More Columns in Python Pandas
How to Get Filename from Path in Python
Plot Graph from CSV Data Using Python Matplotlib
Python Script to Check URL Status
How to Convert CSV to Tab Delimited File in Python
How to Remove HTML Tags from CSV File in Python
How to Split File in Python
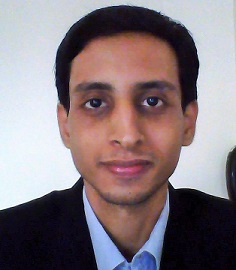
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.