Sometimes you may need to lock a file or directory from your python application or service, to prevent others from modifying it. This is specially required if multiple processes are accessing the same file. Generally, file locks are implemented using operating system tools in Linux and Windows. But sometimes you may need to execute a file lock from within python. In this article, we will learn how to lock file in python. You can use these steps on all Python versions, from within your python-based applications, scripts, services and even websites.
How to Lock File in Python
There are several libraries available to lock files. We will use Portalocker for our purposes. Portalocker provides an easy API for file locking in python. It even supports locking Redis.
Here is the command to install Portalocker.
pip install portalocker
If you use Python<2, use the following command instead to install portalocker.
pip install "portalocker<2"
Once portalocker is installed, you can use it as shown below to lock file test.txt.
import portalocker with portalocker.Lock('test.txt') as fh: fh.write('first instance') ...
We basically call portalocker.Lock() function to lock a file. Once it is locked, other processes will not be able to modify it as long as it is locked.
If you are calling the file lock over a network, then you may need to call os.fsync() before closing the file to actually write all the changes before others can read them.
with portalocker.Lock('test.txt', 'rb+', timeout=60) as fh: # do what you need to do ... # flush and sync to filesystem fh.flush() os.fsync(fh.fileno())
Please note, these locks are of advisory nature in Linux/Unix systems, which is the default setting in these Operating systems. If you want them to be mandatory then you need to mount the filesystems with mand option.
Please note, this solution is not perfect. If your python program terminates abruptly, this file will continue to remain locked and you will need to unlock it manually. Nevertheless, it is a good solution.
if you want to unlock a given file in python, you can use the Unlock command in portalocker.
portalocker.Unlock(fh) OR portalocker.Unlock('test.txt')
In this article, we have learnt how to lock file in python. You can use it to lock files in python.
Also read:
How to Use Boolean Variables in Shell Script
How to Concatenate String Variables in Shell Script
How to Iterate Over Arguments in Shell Script
Bash Script With Optional Arguments
How to Test If Variable is Number in Shell Script
Related posts:
How to Add Text to Image in Python
How to Retrieve POST Request Data in Django
How to Create Python Dictionary from String
How to Check if Key Exists in Python Dictionary
How to Extract Numbers from String in Python
How to Comment in Python
How to Remove All Occurrences of Value from List in Python
How to get length of list in Django Template
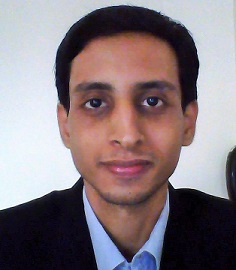
Sreeram has more than 10 years of experience in web development, Python, Linux, SQL and database programming.